Form pattern validation with react-hook-form
23,782
Solution 1
The pattern
attribute on input
only works on submit
in vanilla HTML forms.
If you're using react-hook-form
, it should be in the ref, like this:
<input
name="email"
ref={register({
required: "Required",
pattern: {
value: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i,
message: "invalid email address"
}
})}
/>
please have a check on react-hook-form doc. Additionally for simple form use case you can try cksform library.
Solution 2
If you are using react-hook-form v7 then use this:
<input
placeholder="Email"
{...register('email', {
required: 'Email is required',
pattern: {
value: /^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/,
message: 'Please enter a valid email',
},
})}
type="email"
required
className="input"
/>
{formState.errors.email?.message && (
<FormError errorMessage={formState.errors.email?.message} />
)}
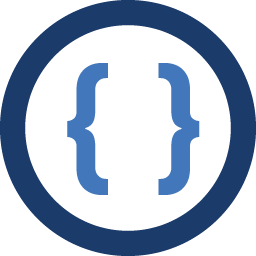
Author by
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
I have been working on a react form and I need to restrict users to put special characters and allow only these ones: [A-Za-z].
I have tried the below code but I am still able to insert inside special characters such as: '♥', '>', '+', etc.
export default Component (props { ... return ( <input pattern={props.pattern} /> ) }
And I am sending it as a prop to my form:
<Component pattern="[A-Za-z]+" />
Can you let me know what I am missing and point out what could be the issue? Many thanks.