Generate all contiguous sequences from an array
15,475
Solution 1
You only have to make 2 changes. The outer loop iterates as much times as the array has elements, this is correct. The first inner loop should use the index of the outer loop as start index (int j = i
), otherwise you always start with the first element. And then change the inner loop break conditon to k <= j
, otherwise i
does not print the last element.
// i is the start index
for (int i = 0; i < items.length; i++)
{
// j is the number of elements which should be printed
for (int j = i; j < items.length; j++)
{
// print the array from i to j
for (int k = i; k <= j; k++)
{
System.out.print(items[k]);
}
System.out.println();
}
}
Solution 2
Tested and working! Try this algorithm.
for(int i=0;i<items.length;i++) {
for(int j=i;j<items.length;j++) {
for(int k=i;k<=j;k++) {
System.out.print(items[k]);
}
System.out.println();
}
}
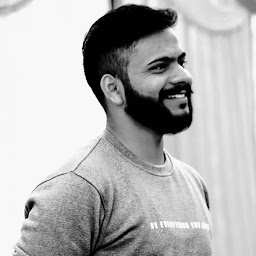
Author by
shubham gupta
Updated on July 25, 2022Comments
-
shubham gupta almost 2 years
There's an array say [1,2,4,5,1]. I need to print all the contiguous sub-arrays from this.
Input:
[1,2,4,5,1]
Output:
1 1,2 1,2,4 1,2,4,5 1,2,4,5,1 2 2,4 2,4,5 2,4,5,1 4 4,5 4,5,1 5 5,1 1
I tried but couldn't get the complete series.
//items is the main array for(int i = 0; i < items.length; i++) { int num = 0; for(int j = 0; j < items.length - i; j++) { for(int k = i; k < j; k++) { System.out.print(items[k]); } System.out.println(); } }
-
kai over 8 yearsNo it does not work. It prints the whole array, then only the last four elements, then the last three elements and so on...
-
kai over 8 yearsThe output is correct, but with much extra spaces between the lines :)
-
Young Song almost 5 yearsUsing loop twice. To get just sum, I suggested using pattern of the subarrays.
-
Ali Akram over 4 yearsis it possible to do in less than 3 loops?
-
kai over 4 years@AliAkram This algorithm has a runtime complexity of O(n²) so you need at least two loops. My code uses only two loops for generating the sequences. The third loop is only for printing. You can replace the inner loop with
System.out.println(Arrays.toString(Arrays.copyOfRange(items, i, j + 1)));
-
chirag soni over 2 years@AliAkram yes possible to use less than 3 loops. Look here: stackoverflow.com/a/70754152/11421611