Generate random sentences in python
Solution 1
The easiest way for your understanding:
import random
nouns = ("puppy", "car", "rabbit", "girl", "monkey")
verbs = ("runs", "hits", "jumps", "drives", "barfs")
adv = ("crazily.", "dutifully.", "foolishly.", "merrily.", "occasionally.")
adj = ("adorable", "clueless", "dirty", "odd", "stupid")
num = random.randrange(0,5)
print nouns[num] + ' ' + verbs[num] + ' ' + adv[num] + ' ' + adj[num]
Solution 2
You can use random.choice
within a list comprehension then concatenate the selected list with join
:
>>> l=[nouns,verbs,adj,adv]
>>> ' '.join([random.choice(i) for i in l])
'girl runs dirty crazily.'
>>> ' '.join([random.choice(i) for i in l])
'monkey hits clueless occasionally.'
Solution 3
For a similar function to the accepted answer that returns all possible permutations:
import random
def make_random_sentence():
nouns = ["puppy", "car", "rabbit", "girl", "monkey"]
verbs = ["runs", "hits", "jumps", "drives", "barfs"]
adv = ["crazily.", "dutifully.", "foolishly.", "merrily.", "occasionally."]
adj = ["adorable", "clueless", "dirty", "odd", "stupid"]
random_entry = lambda x: x[random.randrange(len(x))]
return " ".join([random_entry(nouns), random_entry(verbs), random_entry(adv), random_entry(adj)])
Solution 4
I would do as follows, using random.choice because it's a better choice than random.randrange...
words = (adj, nouns, verb, adv)
sentence = "".join(choice(word) for word in words)
Solution 5
random.choice
is to select a random thing from a list, tuple or similar container.
What the last line does is to select one random thing from each list, and make a new list from the choices. Then that list is printed. And being in a loop, this is done X number of times, whatever number the user inputs.
from random import choice
nouns = ("puppy", "car", "rabbit", "girl", "monkey")
verbs = ("runs", "hits", "jumps", "drives", "barfs")
adv = ("crazily.", "dutifully.", "foolishly.", "merrily.", "occasionally.")
adj = ("adorable", "clueless", "dirty", "odd", "stupid")
# asking user as to how many sentences he would like to generate
for _ in range (int (input ("Enter integer value :"))):
print(list(map(choice, [nouns, verbs, adv, adj])))
Enter integer value :2
['car', 'barfs', 'dutifully.', 'odd']
['rabbit', 'runs', 'occasionally.', 'clueless']
[Program finished]
Related videos on Youtube
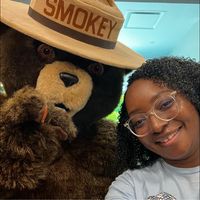
Ashley Glover
Updated on May 04, 2022Comments
-
Ashley Glover 8 months
I'm very new to python and programming!
I need to write a program that has 4 tuples with 5 elements each. One tuple should have verbs, one tuple should have nouns, one tuple should have adjectives, and one tuple should have adverbs. Then I have to use a randomly generated numbers between 0 and 4 to pick one of the elements from each tuple. This is what I have so far:
import random nouns = ("puppy", "car", "rabbit", "girl", "monkey") verbs = ("runs", "hits", "jumps", "drives", "barfs") adv = ("crazily.", "dutifully.", "foolishly.", "merrily.", "occasionally.") adj = ("adorable", "clueless", "dirty", "odd", "stupid") num = random.randrange(0,5) print (num)
Can someone show me what I'm doing wrong?
-
ρss over 7 yearsYou can use that random number to select the corresponding element from each of the tuples. eg:
print nouns[num]
docs.python.org/2/tutorial/… -
abarnert over 7 yearsA better solution would be to use
random.choice(nouns)
… but if your assignment requires you to userandom.randrange
, then you hav eto do it the way @ρss suggests. -
ρss over 7 yearsI agree with @abarnert
-
-
Eric Duminil over 3 yearsNote that with this method, there are only 5 possible sentences, instead of the 625 possible sentences if you pick a random number for each word.
-
Sylvester Kruin about 1 yearNote that with this method, there are only 5 possible sentences, instead of the 625 possible sentences if you pick a random number for each word (same problem with this other answer.