Get all parameters after action in Zend?
Solution 1
As far as I know, you will always get the controller, action and module in the params list as it is part of the default. You could do something like this to remove the three from the array you get:
$url_params = $this->getRequest()->getUserParams();
if(isset($url_params['controller']))
unset($url_params['controller']);
if(isset($url_params['action']))
unset($url_params['action']);
if (isset($url_params['module']))
unset($url_params['module']);
Alternatively as you don't want to be doing that every time you need the list, create a helper to do it for you, something like this:
class Helper_Myparams extends Zend_Controller_Action_Helper_Abstract
{
public $params;
public function __construct()
{
$request = Zend_Controller_Front::getInstance()->getRequest();
$this->params = $request->getParams();
}
public function myparams()
{
if(isset($this->params['controller']))
unset($this->params['controller']);
if(isset($this->params['action']))
unset($this->params['action']);
if (isset($this->params['module']))
unset($this->params['module']);
return $this->params;
}
public function direct()
{
return $this->myparams();
}
}
And you can simply call this from your controller to get the list:
$this->_helper->myparams();
So for example using the url:
http://127.0.0.1/testing/urls/cat/1/page/1/x/111/y/222
And the code:
echo "<pre>";
print_r($this->_helper->myparams());
echo "</pre>";
I get the following array printed:
Array
(
[cat] => 1
[page] => 1
[x] => 111
[y] => 222
)
Solution 2
IMHO this is more elegant and includes changes in action, controller and method keys.
$request = $this->getRequest();
$diffArray = array(
$request->getActionKey(),
$request->getControllerKey(),
$request->getModuleKey()
);
$params = array_diff_key(
$request->getUserParams(),
array_flip($diffArray)
);
Solution 3
How about this?
In controller:
$params = $this->getRequest()->getParams();
unset($params['module'];
unset($params['controller'];
unset($params['action'];
Pretty clunky; might need some isset()
checks to avoid warnings; could jam this segment into its own method or helper. But it would do the job, right?
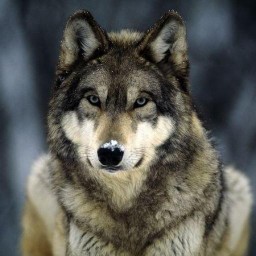
vietean
Updated on June 17, 2022Comments
-
vietean 6 months
When I call a router like below in Zend:
coupon/index/search/cat/1/page/1/x/111/y/222
And inside the controller when I get
$this->_params
, I get an array:array( 'module' => 'coupon', 'controller' => 'index', 'action' => 'search', 'cat' => '1', 'page' => '1', 'x' => '111', 'y' => '222' )
But I want to get only:
array( 'cat' => '1', 'page' => '1', 'x' => '111', 'y' => '222' )
Could you please tell me a way to get the all the
params
just after theaction
? -
Awais Qarni about 11 yearswhy there is a need to write a helper if
$this->getRequest()->getParams()
return the expected value? -
Scoobler about 11 yearsBecause, if you read the posters question, it does not return the expected values, the poster does not want the controller, action and module in the array.
$this->getRequest()->getParams()
WILL return them in its array. Hence removing them withunset
- but if the array is going to be used either several times, or in different controllers, it is easier to use a helper and less code in the controller. -
David Weinraub about 11 yearsSee @Scoobler's answer, which nicely fills in the details to which I allude.