Get integer from Textbox
84,818
Solution 1
You need to parse the value of textbox.Text
which is a string to int
value. You may use int.TryParse, or int.Parse
or Convert.ToInt32
.
TextBox.Text
property is of string
type. You may look at the following sample code.
int.TryParse
This will return true if the parsing is successful and false if it fails.
int value;
if(int.TryParse(textBox1.Text,out value))
{
//parsing successful
}
else
{
//parsing failed.
}
Convert.ToInt32
This may throw an exception if the parsing is unsuccessful.
int value = Convert.ToInt32(textBox1.Text);
int.Parse
int value = int.Parse(textBox1.Text);
Later you can use value
in your if statement like.
if(value > 0)
{
}
else
{
}
Solution 2
Try with this:
int i = int.Parse(textbox1.Text);
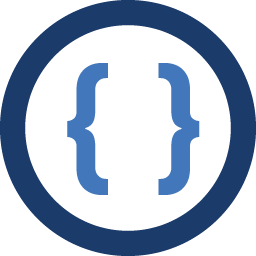
Author by
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I am very new to C# and this question might sound very stupid. I wonder how I'm going get the integer(user's input) from the
textBox1
and use it in if else statement?Please give some examples