Get length of a pointer array in Golang
If you want the length of the thing that v points to, do:
fmt.Println(len(*v))
https://play.golang.org/p/JIWxA2BnoTL
in your second example, you are passing a slice, which contains a length, capacity, and a pointer to the slices first element in the backing array. If you modify an element, it will be modified in both (since the copy of the pointer will still point to the same backing array), but if you append, you are creating a new slice (I believe), but even if not, your original slices length would make it end before the new element regardless.
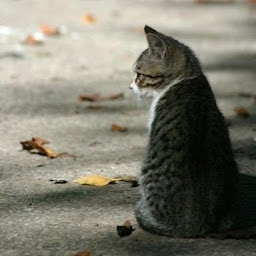
YuanZheng Hu
Updated on June 05, 2022Comments
-
YuanZheng Hu almost 2 years
I am trying to append a vertex into the a slice of vertex by passing the pointer of that slice to the function
AppendVertex
, I know how to get the length of an array by usinglen
function, but is there any way to get the the length of pointer array ?type Vertex struct { X int Y int } func main() { var v []Vertex fmt.Println(len(v)) appendVertex(&v) fmt.Println(len(v)) } func appendVertex(v *[]Vertex) { *v = append(*v, Vertex{1, 1}) fmt.Println(len(v)) }
Result for this one is
prog.go:22:16: invalid argument v (type *[]Vertex) for len
I also did another version by passing the pointer to the slice, however the size of slice did not changed, should't the slice is a reference type structure ? why does the size did not changed here
type Vertex struct { X int Y int } func main() { var v []*Vertex fmt.Println(len(v)) appendVertex(v) fmt.Println(len(v)) } func appendVertex(v []*Vertex) { v = append(v, &Vertex{1, 1}) fmt.Println(len(v)) }
Result for second one is
0 1 0
-
Peter about 6 yearsPossible duplicate of Golang append an item to a slice
-
-
Peter about 6 yearsappend may create a new slice header. That happens if the backing array does not have enough capacity for the new elements. See blog.golang.org/slices for all the gory details.