Get QML root object from anywhere in a code in Qt 5.3
11,414
You don't need the objectName.
Get root element and check that it's a window:
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
QObject *root = engine.rootObjects()[0];
QQuickWindow *window = qobject_cast<QQuickWindow *>(root);
if (!window) {
qFatal("Error: Your root item has to be a window.");
return -1;
}
window->show();
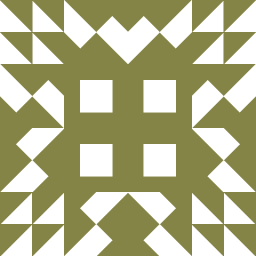
Author by
alexander.sivak
Updated on June 07, 2022Comments
-
alexander.sivak 7 months
- How to retrieve a root object (the code below returns 0,
rootContext()->findChild()
also returns 0) from anywhere in a C++ code (a class method where the class is a registered QML type and the class component definition is a direct child of the root, see objectName) and add a generated QQuickItem at runtime? - There is myclass registered as QML type. How to add a QML
Button
to the scene using the bottommost code?
In the main.qml (fragment)
import QtQuick 2.3 import QtQuick.Window 2.2 Window { objectName: root visible: true width: 360 height: 100 // ... }
myclass.cpp
void myclass::add_hip() { setProperty("hip", 87); QQmlEngine *engine = QtQml::qmlEngine(this); // QObject *root = engine->rootContext()->findChild<QObject*>("womanObj"); QQuickWindow *window = qobject_cast<QQuickWindow *>(root); QObject *wobj = window->findChild<QObject *>("womanObj"); // 1. Define a QML component. QQmlComponent *readHipComp = new QQmlComponent(engine); readHipComp->setData("import QtQuick.Controls 1.2\n\ Button {\n\ anchors.top: addHipBtn.top\n\ anchors.left: addHipBtn.left\n\ anchors.margins: 3\n\ text: \"Hip value\"\n\ onClicked: {\n\ msgDlg.text = myclass.hip\n\ msgDlg.open()\n\ }\ }", QUrl()); // 2. Create the component instance. QQuickItem *readHipBtn = qobject_cast<QQuickItem *>(readHipComp->create()); // 3. Add the instance to the scene. // readHipBtn->setParentItem(qobject_cast<QQuickItem *>(engine->rootContext()->contextObject())); // QObject *root = QtQml::qmlContext(this)->findChild<QQuickItem *>("root"); // readHipBtn->setParent(root); }
UPDATE
extern QQuickWindow *window; void myclass::add_hip() { //... readHipBtn->setParentItem(window->contentItem()); //... }
worked, but 3.) It does not see other main.qml objects.
- How to retrieve a root object (the code below returns 0,