Get the highest values in a hashmap in java
25,076
Solution 1
The first step is to find the highest value at all.
int max = Collections.max(map.values());
Now iterate through all the entries of the map and add to the list keys associated with the highest value.
List<String> keys = new ArrayList<>();
for (Entry<String, Integer> entry : map.entrySet()) {
if (entry.getValue()==max) {
keys.add(entry.getKey());
}
}
If you like the Java 8 Stream API, try the following:
map.entrySet().stream()
.filter(entry -> entry.getValue() == max)
.map(entry -> entry.getKey())
.collect(Collectors.toList());
Solution 2
The response from Nikolas Charalambidis is quite neat, but it may be faster to do it in just one step (iteration), supposing that the input map was much larger:
public static List<String> getKeysWithMaxValue(Map<String, Integer> map){
final List<String> resultList = new ArrayList<String>();
int currentMaxValuevalue = Integer.MIN_VALUE;
for (Map.Entry<String, Integer> entry : map.entrySet()){
if (entry.getValue() > currentMaxValuevalue){
resultList.clear();
resultList.add(entry.getKey());
currentMaxValuevalue = entry.getValue();
} else if (entry.getValue() == currentMaxValuevalue){
resultList.add(entry.getKey());
}
}
return resultList;
}
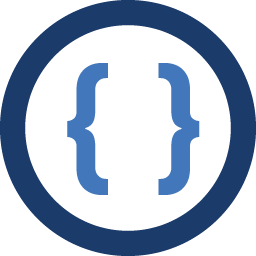
Author by
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I have a HashMap which contains the following values:
Map<String, Integer> map = new HashMap<>(); map.put("name1", 3); map.put("name2", 14); map.put("name3", 4); map.put("name4", 14); map.put("name5", 2); map.put("name6", 6);
How do I get all keys with the highest value? So that I get the following keys in this example:
name2 name4
-
CoolCK about 3 yearselegant solution, nicely explained.