Getting Error: Object doesn't support property or method 'assign'
Solution 1
As others have mentioned, the Object.assign() method is not supported in IE, but there is a polyfill available, just include it "before" your plugin declaration:
if (typeof Object.assign != 'function') {
Object.assign = function(target) {
'use strict';
if (target == null) {
throw new TypeError('Cannot convert undefined or null to object');
}
target = Object(target);
for (var index = 1; index < arguments.length; index++) {
var source = arguments[index];
if (source != null) {
for (var key in source) {
if (Object.prototype.hasOwnProperty.call(source, key)) {
target[key] = source[key];
}
}
}
}
return target;
};
}
From https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign
Test page: http://jsbin.com/pimixel/edit?html,js,output (just remove the polyfill to get the same error you're getting on your page).
Solution 2
@John Doe
I figured out from your comment that you want to implement this in node/react stack. This is very different from original question and you should have asked your own ;)
Anyways, Heres what you need to do...
You can use [es6-object-assign][1]. It is an ES6 Object.assign() "polyfill".
First, in package.json
in your root folder, add es6-object-assign
as a dependency:
"dependencies": {
"es6-object-assign": "^1.0.2",
"react": "^0.12.0",
...
},
Then if you want to use it in node environment use:
require('es6-object-assign').polyfill();
If you are having the issue on front (browser) end...
Add it in your index.html file...
<script src="location_of_node_modules/es6-object-assign/dist/object-assign.min.js"></script>
<script>
window.ObjectAssign.polyfill();
</script>
location_of_node_modules
depends on boilerplate you use, mostly just node_modules
, but sometimes when index.html is in a subdirectory you need to use, ../node_modules
Solution 3
As per the documentation, Object.assign() is a new technology, part of the ECMAScript 2015 (ES6) standard:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign
And it is not supported by IE.
Solution 4
A possible solution for this problem:
Add the script legacy.min.js before the custombox.min.js
source: custombox github project
Solution 5
Since you tagged the question with jQuery you can use the jQuery extend function. No need for a polyfill and it does deep merge as well.
For Example:
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
// Merge object2 into object1
$.extend( object1, object2 );
Result:
{"apple":0,"banana":{"price":200},"cherry":97,"durian":100}
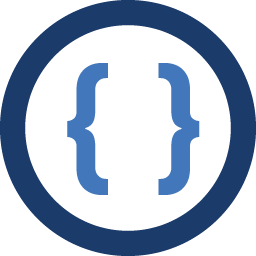
Admin
Updated on July 04, 2020Comments
-
Admin almost 4 years
I am using this jquery popup plugin from this link on my WordPress site. It's working fine on all browsers but giving the following error on IE11.