Getting error while running `react-native run-android`
Solution 1
I just change compileSdkVersion=29 to compileSdkVersion=30 and my issue solved . Its working
Solution 2
As some has mentioned, the problem is that the RN build automatically "upgraded" to androidx.core:core:1.7.0-alpha01, which depends on SDK version 30.
The Fix
The fix is simply to specify android core version via androidXCore in build.gradle
buildscript {
ext {
buildToolsVersion = "29.0.3"
minSdkVersion = 23
compileSdkVersion = 29
targetSdkVersion = 29
ndkVersion = "20.1.5948944"
kotlin_version = "1.5.0"
androidXCore = "1.5.0"
}
How I figured it out
Figuring this out was painful. I grepped for gradle files that would automatically upgrade packages like so
find . -name '*.gradle' -exec grep -H "\.+" {} \;
and found in node_modules/@react-native-community/netinfo/android/build.gradle
the following snippet
def androidXCore = getExtOrInitialValue('androidXCore', null)
if (supportLibVersion && androidXVersion == null && androidXCore == null) {
implementation "com.android.support:appcompat-v7:$supportLibVersion"
} else {
def defaultAndroidXVersion = "1.+"
if (androidXCore == null) {
androidXCore = androidXVersion == null ? defaultAndroidXVersion : androidXVersion
}
implementation "androidx.core:core:$androidXCore"
}
}
Solution 3
Apparently they just broke this today.
You can fix it by adding the following line to your app level build.gradle file (above the android { }
block as a sibling):
configurations.all {
resolutionStrategy { force 'androidx.core:core-ktx:1.6.0' }
}
Finally, the Gradle build was successfully completed. Ref. https://docs.gradle.org/current/dsl/org.gradle.api.artifacts.ResolutionStrategy.html
Solution 4
I got same issue. Just today. When I try to run locally, I got the exact same error. To get it run again, I update the field compileSdkVersion
and targetSdkVersion
in file android > build.gradle from 29 to 30. Then, it can run again. If this answer fits with you, you can go with this way. But, personally, I'm lookin for solution without to change the value compileSdkVersion
and targetSdkVersion
Update: just change the compileSdkVersion
Solution 5
First never use + to define the version of Libraries in Gradle, Try to give the latest version always.
If you are using Core KTX like below in your main Gradle dependencies
implementation "androidx.core:core-ktx:+"
Just replace it to
implementation "androidx.core:core-ktx:1.6.0"
Core and Core-ktx Version 1.7.0 released yesterday(September 1, 2021), which is causing the issues.
https://developer.android.com/jetpack/androidx/releases/core
Related videos on Youtube
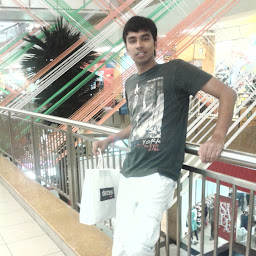
Abhigyan Gaurav
Updated on September 03, 2021Comments
-
Abhigyan Gaurav over 2 years
Whle running my react native code
react-native run-android
getting below error. It was working proper. But after taking fresh pull from git andnpm ci
and after I am running then getting this error.error Failed to install the app. Make sure you have the Android development environment set up: https://reactnative.dev/docs/environment-setup. Error: Command failed: gradlew.bat app:installDebug -PreactNativeDevServerPort=8081 FAILURE: Build failed with an exception. * What went wrong: Execution failed for task ':app:checkDebugAarMetadata'. > A failure occurred while executing com.android.build.gradle.internal.tasks.CheckAarMetadataWorkAction > The minCompileSdk (30) specified in a dependency's AAR metadata (META-INF/com/android/build/gradle/aar-metadata.properties) is greater than this module's compileSdkVersion (android-29). Dependency: androidx.core:core:1.7.0-alpha01. AAR metadata file: C:\Users\gauraab\.gradle\caches\transforms-2\files-2.1\9e02d64f5889006a671d0a7165c73e72\core-1.7.0-alpha01\META-INF\com\android\build\gradle\aar-metadata.properties.
-
ChandrasekarG almost 3 yearsSince everyone is facing it just today, I think this could probably be because of our dependencies. Some update to minor version/patch version broke the android builds probably? In the dependencies, we can try providing an exact stable version number for our dependencies.
-
MagogCZ almost 3 yearssame problem after update Android Studio to 4.2.2
-
Usama Altaf almost 3 yearstry to change the core library with stable version 1.6.0
-
Akza almost 3 years@UsamaAltaf where is the core lib defined? I couldn't found
androidx.core:core:1.7.0-alpha01
in my project
-
-
Akza almost 3 yearsdo you know which dependency it is? Caused I already specified with
implementation 'androidx.appcompat:appcompat:1.1.0-rc01'
. But still got the same error -
ChandrasekarG almost 3 yearsProbably the rc build could have caused this. And in general rc builds are quite buggy. Can you try a stable build?
-
Akza almost 3 yearsdid you use
implementation "com.facebook.react:react-native:+"
? Did you specified it, the version? -
ChandrasekarG almost 3 yearsI don't use react-native. Mine is a native android project. So, you don't have to change it I believe since that did not break things for me.
-
Akza almost 3 yearsI've try to change from
androidx.appcompat:appcompat:1.1.0-rc01
toandroidx.appcompat:appcompat:1.3.0
(like you did). But, still got the error -
ChandrasekarG almost 3 yearsCan you get us the list of all dependencies?
-
Abhigyan Gaurav almost 3 yearscompileSdkVersion=29 to compileSdkVersion=30
-
ChandrasekarG almost 3 yearsLet us continue this discussion in chat.
-
Victor Sheyanov almost 3 years0.64.5 will have all sdk versions updated to 30, so you can use ReactNative upgrade tool and upgrade them all upfront to 30.
-
ChandrasekarG almost 3 yearsDid you try changing the compileSdkVersion=29 to compileSdkVersion=30
-
Rohit Bhargava almost 3 years@ChandrasekarG To most of us, this issue type is being observed in most recent, to be precise since yesterday. TBH, I haven't tried upgrading
compileSdkVersion
, will try and check. -
Gilbert almost 3 yearsSee my answer below: stackoverflow.com/a/68222185/70488
-
Mr Chris almost 3 yearsI was able to build by only adjust 'androidx.appcompat:appcompat:1.3.0', leaving the compileSdkVersion and targetSdkVersion at 29 - thus avoid upgrading the React Native NPM packages.
-
Denis Tsoi over 2 years2021 React-native MVP
-
Rohit Aggarwal over 2 yearsMy release build's size has increased by 2x after this.
-
kojo justine over 2 yearsthis worked for me
-
Nabil Freeman about 2 yearsThank you! This helped me a lot. I also used
./gradlew :app:dependencies > dependencies.txt
(from stackoverflow.com/a/69031232/1395078) which helped me find the random dependency that was forcingcore 1.7
. I had to hunt through several resolutions and eventually found it was Intercom bumpingactivity-compose
between 10.6.2 and 10.6.3. I had10+
so pinned it to 10.6.2. If it's a native package, finding the version to downgrade to is very easy with this repository explorer: mvnrepository.com/artifact/io.intercom.android/… - it shows dependencies.