Global memory management in C++ in stack or heap?
Solution 1
Since I wasn't satisfied with the answers, and hope that the sameer karjatkar wants to learn more than just a simple yes/no answer, here you go.
Typically a process has 5 different areas of memory allocated
- Code - text segment
- Initialized data – data segment
- Uninitialized data – bss segment
- Heap
- Stack
If you really want to learn what is saved where then read and bookmark these:
COMPILER, ASSEMBLER, LINKER AND LOADER: A BRIEF STORY (look at Table w.5)
Anatomy of a Program in Memory
Solution 2
The problem here is the question. Let's assume you've got a tiny C(++ as well, they handle this the same way) program like this:
/* my.c */
char * str = "Your dog has fleas."; /* 1 */
char * buf0 ; /* 2 */
int main(){
char * str2 = "Don't make fun of my dog." ; /* 3 */
static char * str3 = str; /* 4 */
char * buf1 ; /* 5 */
buf0 = malloc(BUFSIZ); /* 6 */
buf1 = malloc(BUFSIZ); /* 7 */
return 0;
}
- This is neither allocated on the stack NOR on the heap. Instead, it's allocated as static data, and put into its own memory segment on most modern machines. The actual string is also being allocated as static data and put into a read-only segment in right-thinking machines.
- is simply a static allocated pointer; room for one address, in static data.
- has the pointer allocated on the stack and will be effectively deallocated when
main
returns. The string, since it's a constant, is allocated in static data space along with the other strings. - is actually allocated exactly like at 2. The
static
keyword tells you that it's not to be allocated on the stack. - ...but
buf1
is on the stack, and - ... the malloc'ed buffer space is on the heap.
- And by the way., kids don't try this at home.
malloc
has a return value of interest; you should always check the return value.
For example:
char * bfr;
if((bfr = malloc(SIZE)) == NULL){
/* malloc failed OMG */
exit(-1);
}
Solution 3
Usually it consumes neither. It tries to allocate them in a memory segment which is likely to remain constant-size for the program execution. It might be bss, stack, heap or data.
Solution 4
Global memory is pre-allocated in a fixed memory block, or on the heap, depending on how it is allocated by your application:
byte x[10]; // pre-allocated by the compiler in some fixed memory block
byte *y
main()
{
y = malloc(10); // allocated on the heap
}
EDIT:
The question is confusing: If I allocate a data structure globally in a C++ application , does it consume stack memory or heap memory ?
"allocate"? That could mean many things, including calling malloc(). It would have been different if the question was "if I declare and initialize a data structure globally".
Many years ago, when CPUs were still using 64K segments, some compilers were smart enough to dynamically allocate memory from the heap instead of reserving a block in the .data segment (because of limitations in the memory architecture).
I guess I'm just too old....
Solution 5
Neither. It is .data section.
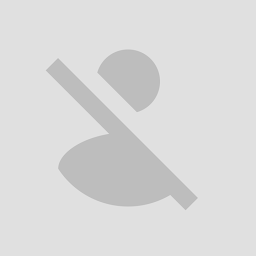
Alex Carman
Updated on July 05, 2022Comments
-
Alex Carman almost 2 years
If I declare a data structure globally in a C++ application , does it consume stack memory or heap memory ?
For eg
struct AAA { .../.../. ../../.. }arr[59652323];
-
Philippe Leybaert almost 15 yearsIt depends if the global memory was allocated inline or alllocated dynamically from the application
-
Philippe Leybaert almost 15 yearsglobal memory is never allocated on the stack. The stack is only used for local variables and parameters
-
EFraim almost 15 yearsIf a memory was allocated dynamically it is not global (in a sense of global variable)
-
Alex Carman almost 15 yearsBy editing the boot.ini file we can extend the virtual memory to 3GB . Like wise is there any setting for the memory segment ?
-
EFraim almost 15 yearsThen in what sense it is global, if it is not in scope of all the program?!
-
user128026 almost 15 yearsstack variables are "destroyed" when the function returns
-
Philippe Leybaert almost 15 yearsThat would be pointless, because the size of the statically allocated memory can never change
-
EFraim almost 15 yearsThe malloced buffer space has nothing to do with global variables. Only the pointers are global. Please to not confuse the people further.
-
Falaina almost 15 yearsI'd have to agree with EFraim. The actual global variable is going to be located in the .data/.bss sections. Sure in the case of a pointer, it may eventually point to dynamically allocated memory, but I wouldn't say the pointer itself is dynamically allocated.
-
Don Johe almost 15 yearsIt sais "allocated on the heap" and that's pretty correct. Unless this question is flagged "novice" or "beginner" this should be a sufficient reminder to what is happening.
-
Philippe Leybaert almost 15 yearsWe're talking about allocated memory which is globally accessible through a global variable. In fact, the compiler can even choose to allocate a pre-allocated block of memory on the heap instead of in a fixed memory block (if the block is too large)
-
Alex Carman almost 15 yearsIf the global variable is going to be located in the data/.bss sections will it show up in the heap memory consumption . I mean will it record its memory consumption under virtual memory ?
-
Philippe Leybaert almost 15 yearsAnother point worth noting is that ".data" sections are EXE only (so Microsoft-stuff). There are of course similar structures on other OS's , but the question was not specifically about PC development
-
EFraim almost 15 years@Don: No. The global thing is the pointer, and not the memory it is pointing to. You can handle the memory the way you want. Neither it is there to stay for all the run. You can even point it at stack sometimes.
-
EFraim almost 15 years@Philippe - the point is that the data pointed to by global pointer cannot be considered global. It can even change during program execution (different functions might reset the global pointer to whatever place they want)
-
Alex Carman almost 15 yearsDoes that mean the Uninitialized data - bss and the Initialized - data are a part of the heap ?
-
Milan almost 15 yearsNo, they are not a part of the heap, they are in different areas as was written in my answer (the 5 different areas). The heap and stack occupy the virtual memory above the text and data segments.
-
Philippe Leybaert almost 15 yearsIf there is one lesson to be learned from this, it's that you should avoid answering questions where the exact meaning of the question is unclear. My answer is not wrong, it's just that some people think that their interpretation of a word is enough to vote down everything that doesn't support their view. Even now, 10 hours after the question was asked, it's still not clear what the OP meant.
-
Alex Carman almost 15 yearsYes that's my mistake in framing the question . I have edited it now
-
quark almost 15 yearsThe important point is that the bss and data segments are allocated when the program is first loaded into memory, and their size does not change while it runs. The contents of the heap by contrast are volatile and change throughout the run, as dynamic memory operations are performed.
-
Charlie Martin almost 15 yearsOh, don't be silly. The questioner clearly wasn't clear on what went where, so I wrote an answer that was directed to improving his understanding.
-
EFraim almost 13 years@Philippe: .data sections are also not .EXE only.
-
danijar over 9 yearsI thought the idea of letting the stack grow downwards and letting the heap grow upwards was so that they can use the available memory in any ratio. However, isn't that prevented by loading the dynamic libraries in between?
-
philx_x almost 6 yearsdoes initializing a pointer to NULL go in the data or bss segment?
route_t* tblhead = NULL;