Google Maps v2 Error on use of setMyLocationEnabled
Solution 1
getmap()
is returning null. That means the map is not ready. Either because the fragment is not ready or your are running on a device without Google Play services available. See getMap for more info.
Solution 2
I was getting something simular due to the Google Play Services API being missing from the device. If you use:
GooglePlayServicesUtil.isGooglePlayServicesAvailable(Context)
It should return ConnectionResult.SUCCESS if available. if it returns
ConnectionResult.SERVICE_MISSING
ConnectionResult.SERVICE_VERSION_UPDATE_REQUIRED
ConnectionResult.SERVICE_DISABLED
you can prompt the user to download it using
GooglePlayServicesUtil.getErrorDialog()
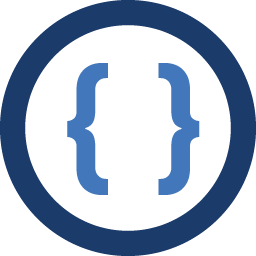
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am not sure were I went wrong, getting a
nullPointerException
on usinggooglemap.setMyLocationEnabled(true)
in Google Map v2 example.MainActivity.java File:
package com.example.locationgooglemapsv2; import java.io.IOException; import java.util.List; import android.content.Context; import android.location.Address; import android.location.Criteria; import android.location.Geocoder; import android.location.Location; import android.location.LocationListener; import android.location.LocationManager; import android.os.AsyncTask; import android.os.Bundle; import android.support.v4.app.FragmentActivity; import android.view.Menu; import android.widget.RadioGroup; import android.widget.RadioGroup.OnCheckedChangeListener; import com.google.android.gms.maps.CameraUpdateFactory; import com.google.android.gms.maps.GoogleMap; import com.google.android.gms.maps.GoogleMap.OnMapClickListener; import com.google.android.gms.maps.SupportMapFragment; import com.google.android.gms.maps.model.LatLng; import com.google.android.gms.maps.model.MarkerOptions; public class MainActivity extends FragmentActivity implements LocationListener { GoogleMap googleMap; MarkerOptions markerOptions; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Getting reference to the SupportMapFragment of activity_main.xml SupportMapFragment fm = (SupportMapFragment) getSupportFragmentManager().findFragmentById(R.id.map); // Getting GoogleMap object from the fragment googleMap = fm.getMap(); // Enabling MyLocation Layer of Google Map googleMap.setMyLocationEnabled(true); /*======== MAP TYPE ==========*/ googleMap.setMapType(GoogleMap.MAP_TYPE_NORMAL); RadioGroup rgViews = (RadioGroup) findViewById(R.id.rg_views); rgViews.setOnCheckedChangeListener(new OnCheckedChangeListener() { @Override public void onCheckedChanged(RadioGroup group, int checkedId) { // TODO Auto-generated method stub switch(checkedId){ case R.id.normal: googleMap.setMapType(GoogleMap.MAP_TYPE_NORMAL); break; case R.id.satellite:googleMap.setMapType(GoogleMap.MAP_TYPE_SATELLITE); break; case R.id.terrain: googleMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN); break; } } }); /*======== MARKER AND ONCLICK ==========*/ googleMap.setOnMapClickListener(new OnMapClickListener() { @Override public void onMapClick(LatLng latlng) { // Creating a marker MarkerOptions markerOption = new MarkerOptions(); // Setting the position for the marker markerOption.position(latlng); // Setting the title for the marker. // This will be displayed on taping the marker //markerOption.title(latlng.latitude + " : " + latlng.longitude); // Clears the previously touched position googleMap.clear(); // Animating to the touched position googleMap.animateCamera(CameraUpdateFactory.newLatLng(latlng)); // Placing a marker on the touched position googleMap.addMarker(markerOption); // Adding Marker on the touched location with address new ReverseGeocodingTask(getBaseContext()).execute(latlng); } }); /*======== LOCATION ==========*/ // Getting LocationManager object from System Service LOCATION_SERVICE LocationManager locationManager = (LocationManager) getSystemService(LOCATION_SERVICE); // Creating a criteria object to retrieve provider Criteria criteria = new Criteria(); // Getting the name of the best provider String provider = locationManager.getBestProvider(criteria, true); // Getting Current Location Location location = locationManager.getLastKnownLocation(provider); if(location!=null){ onLocationChanged(location); } locationManager.requestLocationUpdates(provider, 20000, 0, this); } @Override public void onLocationChanged(Location location) { // TODO Auto-generated method stub double lat = location.getLatitude(); double lng = location.getLongitude(); //--maps LatLng latlng = new LatLng(lat, lng); googleMap.moveCamera(CameraUpdateFactory.newLatLng(latlng)); googleMap.animateCamera(CameraUpdateFactory.zoomTo(10)); } @Override public void onProviderDisabled(String provider) { // TODO Auto-generated method stub } @Override public void onProviderEnabled(String provider) { // TODO Auto-generated method stub } @Override public void onStatusChanged(String provider, int status, Bundle extras) { // TODO Auto-generated method stub } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.activity_main, menu); return true; } /*======== REVERSE GEO CODING ==========*/ class ReverseGeocodingTask extends AsyncTask<LatLng, Void, String>{ Context mContext; public ReverseGeocodingTask( Context con) { super(); this.mContext = con; } @Override protected String doInBackground(LatLng... params) { // TODO Auto-generated method stub Geocoder geocoder = new Geocoder(mContext); double lat = params[0].latitude; double lng = params[0].longitude; List<Address> addresses = null; String actualAdress = ""; try { addresses = geocoder.getFromLocation(lat, lng, 1); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } if(addresses != null && addresses.size() > 0){ Address address = addresses.get(0); actualAdress = String.format("%s, %s, %s", address.getMaxAddressLineIndex() > 0 ? address.getAddressLine(0) : "", address.getLocality(), address.getCountryName()); } return actualAdress; } @Override protected void onPostExecute(String result) { // TODO Auto-generated method stub super.onPostExecute(result); // Setting the title for the marker. // This will be displayed on taping the marker markerOptions.title(result); // Placing a marker on the touched position googleMap.addMarker(markerOptions); } } }
Manifest File:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.locationgooglemapsv2" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="16" /> <permission android:name="com.example.locationgooglemapsv2.permission.MAPS_RECIEVE" android:protectionLevel="signature" > </permission> <uses-permission android:name="com.example.locationgooglemapsv2.permission.MAPS_RECIEVE" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" /> <uses-feature android:glEsVersion="0x00020000" android:required="true"/> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.locationgooglemapsv2.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <meta-data android:name="com.google.android.maps.v2.API_KEY" android:value="API_KEY" /> </application> </manifest>
Error Log:
01-11 21:11:56.282: E/AndroidRuntime(327): FATAL EXCEPTION: main 01-11 21:11:56.282: E/AndroidRuntime(327): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.locationgooglemapsv2/com.example.locationgooglemapsv2.MainActivity}: java.lang.NullPointerException 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2663) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2679) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread.access$2300(ActivityThread.java:125) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:2033) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.os.Handler.dispatchMessage(Handler.java:99) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.os.Looper.loop(Looper.java:123) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread.main(ActivityThread.java:4627) 01-11 21:11:56.282: E/AndroidRuntime(327): at java.lang.reflect.Method.invokeNative(Native Method) 01-11 21:11:56.282: E/AndroidRuntime(327): at java.lang.reflect.Method.invoke(Method.java:521) 01-11 21:11:56.282: E/AndroidRuntime(327): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:868) 01-11 21:11:56.282: E/AndroidRuntime(327): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:626) 01-11 21:11:56.282: E/AndroidRuntime(327): at dalvik.system.NativeStart.main(Native Method) 01-11 21:11:56.282: E/AndroidRuntime(327): Caused by: java.lang.NullPointerException 01-11 21:11:56.282: E/AndroidRuntime(327): at com.example.locationgooglemapsv2.MainActivity.onCreate(MainActivity.java:46) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1047) 01-11 21:11:56.282: E/AndroidRuntime(327): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2627) 01-11 21:11:56.282: E/AndroidRuntime(327): ... 11 more
BTW the source is a good example for those who are trying to play with
google map v2
and is well commented.Any help regarding the error is appreciated.
Thanks in advance.
-
Spons almost 11 yearsIs there a way to remove the googleplayservice to test this workflow?
-
azza50 almost 11 yearsI think its removable from your device through the applications menu.