grep return 0 if no match
Solution 1
The actual answer to this question is to add || true
to the end of the command, e.g.:
echo thing | grep x || true
This will still output a 0 return code.
Solution 2
You are processing the return value incorrectly.
value=$( grep -ic "210.64.203" /var/logs )
sets value
to the output of the grep, not to its return code.
After executing a command the exit code it stored in $?
, but you usually don't need it.
if grep -ic "210.64.203" /var/logs
then echo "Found..."
else echo "not found"
fi
If you want the value, then test for content.
rec="$( grep -ic "210.64.203" /var/logs )"
if [ -n "$rec" ] ; then echo found; fi
Or if using bash
,
if [[ "$rec" ]] ; then echo found; fi
though I prefer to be explicit -
if [[ -n "$rec" ]] ; then echo found; fi
Solution 3
[user@host ~]$ cat ~/mylogfile.txt
no secrets here
[user@host ~]$ grepnot(){ ! grep $1 $2; return $?;}
[user@host ~]$ grepnot password mylogfile.txt
[user@host ~]$ echo $?
0
[user@host ~]$ grepnot secret mylogfile.txt
no secrets here
[user@host ~]$ echo $?
1
Note: I'm using 'grepnot' in a Jenkins pipeline. My previous answer used "1 - $?" to reverse the return code. But that solution still caused grep failure in the pipeline. The above solution (which borrows from a previous "!" answer) works with the pipeline.
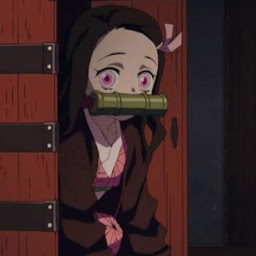
julian lee
Updated on June 04, 2022Comments
-
julian lee almost 2 years
Is there any bash script which can return me a result=true with command grep?
Example: There are 1000 records of 103.12.88 in my CF logs. Can I do a grep 103.12.88 if detect 1 or more results then print/output result show me either YES or True
-
William Pursell over 4 yearsRather than
if [ ! "$rec" = "" ]
, it is generally cleaner to writeif [ -n "$rec" ]
-
Paul Hodges over 4 yearsEdited. I always use that with
[[
inbash
, but since I so seldom use[
it didn't occur to me to try it while I was testing. Thanks. -
Paul Hodges almost 4 yearsNo it doesn't. That forces a 0 return, but generates no output at all. Try
ret=$( echo thing | grep x || true )
vsret=$( echo thing | grep -q x; echo $?; )
The former leaves$ret
empty. The latter loads the exit code. Maybe you meantecho thing | grep x && echo true
? -
sebcoe almost 4 yearsNope. I want to always return a 0 return code regardless of whether grep matches or not (which in the example it won't).
-
Paul Hodges almost 4 yearsexit code and output are separate things. OP says
Can I do a grep 103.12.88 if detect 1 or more results then print/output result show me either YES or True
, which is output. Your solution is fine, it just doesn't do what the poorly worded OP asked. -
lonix about 3 yearsThis doesn't work - there is no
not
in bash, and the second line does indeed print. Are you using a different shell? -
Telmo Trooper about 3 yearsNow that you mentioned it, it seems
not
is a program that comes withLLVM
(which I already had installed). I'll try to gather up more information to update my answer, but thank you for noticing the issue. -
lonix about 3 yearsWas wondering why it didn't work for me :)
-
ob-ivan almost 3 yearsA single exclamation mark
!
does what the reponder'snot
program does: it 'inverts' the status code. So! true
exits with the status code 1 and! false
exits with the status code 0.