Grub 'incompatible license' error
Solution 1
Update2:
Forgot you posted the tar ball. Too bad. Anyhow, did a test on your .mod
files
by using the below code and:
./grum_lic_test32 evan_teitelman/boot/grub/i386-pc/*.mod
which yielded the following error:
...
bufio.mod License: LICENSE=GPLv3+ OK
cacheinfo.mod License: LICENSE=NONE_FOUND ERR
cat.mod License: LICENSE=GPLv3+ OK
chain.mod License: LICENSE=GPLv3+ OK
...
but that file is identical with the one from Archlinux download, so it should not be an issue. In other words, was not the cause.
Also, first now, notice you have installed LILO, – and guess by that the case is closed. If not there is always the question about GPT and BIOS + other issues. Did you install it the first time? Can be that there was some tweak involved on first install that reinstall of GRUB did not fix.
Update1: OK. Fixed. Should work for both 32 and 64-bit ELF
's.
When GRUB
get to the phase of loading modules it check for license embedded
in ELF
file for each module. If non valid is found the module is ignored –
and that specific error is printed. Could be one or more modules are corrupted.
If it is an essential module everything would go bad. Say e.g part_gpt.mod
or part_msdos.mod
.
Accepted licenses are GPLv2+
, GPLv3
and GPLv3+
.
It could of course be other reasons; but one of many could be corrupted module file(s).
It seems like the modules are valid ELF
files as they are validated as such
before the license test. As in: if ELF
test fail license test is not executed.
Had another issue with modules where I needed to check for various, have
extracted parts of that code and made it into a quick license tester. You could
test each *.mod
file in /boot/grub/*
to see which one(s) are corrupt.
This code does not validate ELF
or anything else. Only try to locate license
string and check that. Further it is only tested under i386/32-bit. The original
code where it is extracted from worked for x86-64 as well – but here a lot is stripped and hacked so I'm not sure of the result. If it doesn't work under 64-bit it should most likely only print License: LICENSE=NONE_FOUND
.
(As noted in edit above I have now tested for 32 and 64-bit, Intel.)
As a separate test then would be to do something like:
xxd file.mod | grep -C1 LIC
Not the most beautiful code – but as a quick and dirty check.
(As in; you could try.)
Compile instructions e.g.:
gcc -o grub_lic_test32 source.c # 32-bit variant
gcc -o grub_lic_test64 source.c -DELF64 # 64-bit variant
Run:
./grub_lic_test32 /path/to/mods/*.mod
Prints each file and license, eg:
./grub_lic_test32 tar.mod gettext.mod pxe.mod
tar.mod License: LICENSE=GPLv1+ BAD
gettext.mod License: LICENSE=GPLv3+ OK
pxe.mod License: LICENSE=GPLv3+ OK
Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <errno.h>
#ifdef ELF64
struct ELF_hdr
{
unsigned char dummy0[16];
uint32_t dummy1[6];
uint64_t sh_off;
uint16_t dummy2[5];
uint16_t sh_entsize;
uint16_t sh_num;
uint16_t sh_strndx;
};
struct ELF_sect_hdr
{
uint32_t sh_name;
uint32_t dummy0[5];
uint64_t sh_offset;
};
#else
struct ELF_hdr
{
unsigned char dummy0[16];
uint32_t dummy1[4];
uint32_t sh_off;
uint16_t dummy2[5];
uint16_t sh_entsize;
uint16_t sh_num;
uint16_t sh_strndx;
};
struct ELF_sect_hdr
{
uint32_t sh_name;
uint32_t dummy[3];
uint32_t sh_offset;
};
#endif
enum {
ERR_FILE_OPEN = 1,
ERR_FILE_READ,
ERR_MEM,
ERR_BAD_LICENSE,
ERR_ELF_SECT_CORE_BREACH
};
int file_size(FILE *fh, size_t *fs)
{
size_t cp;
cp = ftell(fh);
fseek(fh, 0, SEEK_END);
*fs = ftell(fh);
fseek(fh, cp, SEEK_SET);
return 0;
}
static const char *valid_licenses[] = {
"LICENSE=GPLv2+",
"LICENSE=GPLv3",
"LICENSE=GPLv3+",
NULL
};
int grub_check_license(struct ELF_hdr *e)
{
struct ELF_sect_hdr *s;
const char *txt;
const char *lic;
unsigned i, j = 0;
s = (struct ELF_sect_hdr *)
((char *) e + e->sh_off + e->sh_strndx * e->sh_entsize);
txt = (char *) e + s->sh_offset;
s = (struct ELF_sect_hdr *) ((char *) e + e->sh_off);
for (i = 0; i < e->sh_num; ++i) {
if (strcmp (txt + s->sh_name, ".module_license") == 0) {
lic = (char*) e + s->sh_offset;
if (j)
fprintf(stdout, "%25s", "");
fprintf(stdout, "License: %-25s ", lic);
for (j = 0; valid_licenses[j]; ++j) {
if (!strcmp (lic, valid_licenses[j])) {
fprintf(stdout, "OK\n");
return 0;
}
}
fprintf(stdout, "BAD\n");
}
s = (struct ELF_sect_hdr *) ((char *) s + e->sh_entsize);
}
if (!j)
fprintf(stdout, "License: %-25s ERR\n", "LICENSE=NONE_FOUND");
return ERR_BAD_LICENSE;
}
int grub_check_module(void *buf, size_t size, int verbose)
{
struct ELF_hdr *e = buf;
/* Make sure that every section is within the core. */
if (e->sh_off + e->sh_entsize * e->sh_num > size) {
fprintf(stderr, "ERR: Sections outside core\n");
if (verbose)
fprintf(stderr,
" %*s: %u bytes\n"
#ifdef ELF64
" %*s %u < %llu\n"
" %*s: %llu\n"
#else
" %*s %u < %u\n"
" %*s: %u\n"
#endif
" %*s: %u\n"
" %*s: %u\n"
,
-25, "file-size", size,
-25, "",
size, e->sh_off + e->sh_entsize * e->sh_num,
-25, "sector header offset", e->sh_off,
-25, "sector header entry size", e->sh_entsize,
-25, "sector header num", e->sh_num
);
return ERR_ELF_SECT_CORE_BREACH;
}
return grub_check_license(e);
}
int grub_check_module_file(const char *fn, int verbose)
{
FILE *fh;
void *buf;
size_t fs;
int eno;
char *base_fn;
if (!(base_fn = strrchr(fn, '/')))
base_fn = (char*)fn;
else
++base_fn;
fprintf(stderr, "%-25s ", base_fn);
if (!(fh = fopen(fn, "rb"))) {
fprintf(stderr, "ERR: Unable to open `%s'\n", fn);
perror("fopen");
return ERR_FILE_OPEN;
}
file_size(fh, &fs);
if (!(buf = malloc(fs))) {
fprintf(stderr, "ERR: Memory.\n");
fclose(fh);
return ERR_MEM;
}
if (fread(buf, 1, fs, fh) != fs) {
fprintf(stderr, "ERR: Reading `%s'\n", fn);
perror("fread");
free(buf);
fclose(fh);
return ERR_FILE_READ;
}
fclose(fh);
eno = grub_check_module(buf, fs, verbose);
free(buf);
return eno;
}
int main(int argc, char *argv[])
{
int i = 1;
int eno = 0;
int verbose = 0;
if (argc > 1 && argv[1][0] == '-' && argv[1][1] == 'v') {
verbose = 1;
++i;
}
if (argc - i < 1) {
fprintf(stderr, "Usage: %s [-v] <FILE>[, FILE[, ...]]\n", argv[0]);
return 1;
}
for (; i < argc; ++i) {
eno |= grub_check_module_file(argv[i], verbose);
if (eno == ERR_MEM)
return eno;
}
return eno;
}
Solution 2
Take a look at this thread over on ubuntugeek.com titled: Boot-Repair – Simple tool to repair frequent boot problems. This tool may be able to help repair what's wrong with your HDD. Looking at the list of features this seems like the easiest way to try and repair your boot partition without getting your hands too dirty.
Related videos on Youtube
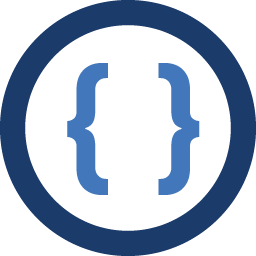
Admin
Updated on September 18, 2022Comments
-
Admin over 1 year
The other day, I was using a laptop for general desktop use when its keyboard began to act up. Most of the keys on the keyboard's right side stopped working entirely and key combinations such as Ctrlu made characters appear that should not have appeared. The backspace key exhibited the strangest behavior; it was somehow able to cause the deletion characters in the shell prompt.
I was unable to reboot the computer cleanly so I did a hard shutdown. When I turned the computer on again, I received this message from Grub:
GRUB loading. Welcome to GRUB! incompatible license Aborted. Press any key to exit.
I pressed the any key and Grub responded with
Operating System Not Found.
Pressing another key causes the first message to appear again. Pressing another key after that causes the second message to appear... and so on.
If I leave the laptop on for a few minutes, its fan speeds up significantly as if the laptop is running a CPU-intensive program.
I took the hard drive out of the laptop, mounted it on a server, and looked around. I saw nothing strange in
/boot
.The laptop is running Arch Linux. The drive is partitioned with GPT. The laptop works fine with a hard drive from another machine. And other machines do not work with the laptop's hard drive.
I am not certain that the keyboard issues are directly related to the Grub issues.
What could be causing the problems that I am having? Or, what should I do to find out or narrow down the list of potential causes?
Just in case it's relevant, here (removed) is a tarball with
/boot
and/etc/grub.d
and here is my Grub configuration:# # DO NOT EDIT THIS FILE # # It is automatically generated by grub-mkconfig using templates # from /etc/grub.d and settings from /etc/default/grub # ### BEGIN /etc/grub.d/00_header ### insmod part_gpt insmod part_msdos if [ -s $prefix/grubenv ]; then load_env fi set default="0" if [ x"${feature_menuentry_id}" = xy ]; then menuentry_id_option="--id" else menuentry_id_option="" fi export menuentry_id_option if [ "${prev_saved_entry}" ]; then set saved_entry="${prev_saved_entry}" save_env saved_entry set prev_saved_entry= save_env prev_saved_entry set boot_once=true fi function savedefault { if [ -z "${boot_once}" ]; then saved_entry="${chosen}" save_env saved_entry fi } function load_video { if [ x$feature_all_video_module = xy ]; then insmod all_video else insmod efi_gop insmod efi_uga insmod ieee1275_fb insmod vbe insmod vga insmod video_bochs insmod video_cirrus fi } if [ x$feature_default_font_path = xy ] ; then font=unicode else insmod part_gpt insmod ext2 set root='hd0,gpt1' if [ x$feature_platform_search_hint = xy ]; then search --no-floppy --fs-uuid --set=root --hint-bios=hd0,gpt1 --hint-efi=hd0,gpt1 --hint-baremetal=ahci0,gpt1 d44f2a2f-c369-456b-81f1-efa13f9caae2 else search --no-floppy --fs-uuid --set=root d44f2a2f-c369-456b-81f1-efa13f9caae2 fi font="/usr/share/grub/unicode.pf2" fi if loadfont $font ; then set gfxmode=auto load_video insmod gfxterm set locale_dir=$prefix/locale set lang=en_US insmod gettext fi terminal_input console terminal_output gfxterm set timeout=5 ### END /etc/grub.d/00_header ### ### BEGIN /etc/grub.d/10_linux ### menuentry 'Arch GNU/Linux, with Linux PARA kernel' --class arch --class gnu-linux --class gnu --class os $menuentry_id_option 'gnulinux-PARA kernel-true-d44f2a2f-c369-456b-81f1-efa13f9caae2' { load_video set gfxpayload=keep insmod gzio insmod part_gpt insmod ext2 set root='hd1,gpt1' if [ x$feature_platform_search_hint = xy ]; then search --no-floppy --fs-uuid --set=root --hint-bios=hd1,gpt1 --hint-efi=hd1,gpt1 --hint-baremetal=ahci1,gpt1 b4fbf4f8-303c-49bd-a52f-6049e1623a26 else search --no-floppy --fs-uuid --set=root b4fbf4f8-303c-49bd-a52f-6049e1623a26 fi echo 'Loading Linux PARA kernel ...' linux /boot/vmlinuz-linux-PARA root=UUID=d44f2a2f-c369-456b-81f1-efa13f9caae2 ro quiet echo 'Loading initial ramdisk ...' initrd /boot/initramfs-linux-PARA.img } menuentry 'Arch GNU/Linux, with Linux core repo kernel' --class arch --class gnu-linux --class gnu --class os $menuentry_id_option 'gnulinux-core repo kernel-true-d44f2a2f-c369-456b-81f1-efa13f9caae2' { load_video set gfxpayload=keep insmod gzio insmod part_gpt insmod ext2 set root='hd1,gpt1' if [ x$feature_platform_search_hint = xy ]; then search --no-floppy --fs-uuid --set=root --hint-bios=hd1,gpt1 --hint-efi=hd1,gpt1 --hint-baremetal=ahci1,gpt1 b4fbf4f8-303c-49bd-a52f-6049e1623a26 else search --no-floppy --fs-uuid --set=root b4fbf4f8-303c-49bd-a52f-6049e1623a26 fi echo 'Loading Linux core repo kernel ...' linux /boot/vmlinuz-linux root=UUID=d44f2a2f-c369-456b-81f1-efa13f9caae2 ro quiet echo 'Loading initial ramdisk ...' initrd /boot/initramfs-linux.img } menuentry 'Arch GNU/Linux, with Linux core repo kernel (Fallback initramfs)' --class arch --class gnu-linux --class gnu --class os $menuentry_id_option 'gnulinux-core repo kernel-fallback-d44f2a2f-c369-456b-81f1-efa13f9caae2' { load_video set gfxpayload=keep insmod gzio insmod part_gpt insmod ext2 set root='hd1,gpt1' if [ x$feature_platform_search_hint = xy ]; then search --no-floppy --fs-uuid --set=root --hint-bios=hd1,gpt1 --hint-efi=hd1,gpt1 --hint-baremetal=ahci1,gpt1 b4fbf4f8-303c-49bd-a52f-6049e1623a26 else search --no-floppy --fs-uuid --set=root b4fbf4f8-303c-49bd-a52f-6049e1623a26 fi echo 'Loading Linux core repo kernel ...' linux /boot/vmlinuz-linux root=UUID=d44f2a2f-c369-456b-81f1-efa13f9caae2 ro quiet echo 'Loading initial ramdisk ...' initrd /boot/initramfs-linux-fallback.img } ### END /etc/grub.d/10_linux ### ### BEGIN /etc/grub.d/20_linux_xen ### ### END /etc/grub.d/20_linux_xen ### ### BEGIN /etc/grub.d/20_memtest86+ ### menuentry "Memory test (memtest86+)" --class memtest86 --class gnu --class tool { insmod part_gpt insmod ext2 set root='hd1,gpt1' if [ x$feature_platform_search_hint = xy ]; then search --no-floppy --fs-uuid --set=root --hint-bios=hd1,gpt1 --hint-efi=hd1,gpt1 --hint-baremetal=ahci1,gpt1 b4fbf4f8-303c-49bd-a52f-6049e1623a26 else search --no-floppy --fs-uuid --set=root b4fbf4f8-303c-49bd-a52f-6049e1623a26 fi linux16 ($root)/boot/memtest86+/memtest.bin } ### END /etc/grub.d/20_memtest86+ ### ### BEGIN /etc/grub.d/30_os-prober ### ### END /etc/grub.d/30_os-prober ### ### BEGIN /etc/grub.d/40_custom ### # This file provides an easy way to add custom menu entries. Simply type the # menu entries you want to add after this comment. Be careful not to change # the 'exec tail' line above. ### END /etc/grub.d/40_custom ### ### BEGIN /etc/grub.d/41_custom ### if [ -f ${config_directory}/custom.cfg ]; then source ${config_directory}/custom.cfg elif [ -z "${config_directory}" -a -f $prefix/custom.cfg ]; then source $prefix/custom.cfg; fi ### END /etc/grub.d/41_custom ###
Update
After installing LILO last night, the computer booted just fine at least once. When I booted the computer this morning, I was faced with a kernel panic:
Initramfs unpacking failed: junk in compressed archive Kernel panic - not syncing: VFS: Unable to mount root fs on unknown-block(8,1) Pid: 1, comm: swapper/0 Not tainted 3.8.7-1-ARCH #1 Call Trace: ...
Here is a picture of the kernel panic.
Update 2
I reinstalled LILO and no longer receive the kernel panic on boot.
-
Admin about 11 yearsThis may well be a hardware problem. First thing to try: yank out the battery and the power plug, wait a few seconds, try again. Second thing: do a memory test, let it stew for a few hours.
-
Admin about 11 yearsI've tried removing all sources of power. I'll run a memory test right now.
-
Admin about 11 yearsI also tried reinstalling Grub with no effect. I'm going to install syslinux.
-
Admin about 11 yearsI ended up installing LILO, which does not seem to be having any problems. The memory test did not find any errors and I don't see any keyboard issues (terminal or shell issues, perhaps?) Is it possible that the hard shutdown caused the Grub issues?
-
Admin about 11 yearsNow I am receiving a kernel panic on boot (see update to question.)
-
Admin about 11 yearsI reinstalled LILO and I no longer receive the kernel panic on boot.
-
-
Florian Bidabé about 11 yearsI don't recall tweaking my Grub install. Thank you for spending the time to put together that module testing code.
-
Admin about 11 yearsAfter seeing the image you posted, I reinstalled Grub. Unfortunately, the reinstall seemed to have no effect.
-
Florian Bidabé about 11 yearsYour answer didn't directly answer my original question so I modified my question a bit. Once this question is eligible for bounty, I will send another 50 reputation your way to repay you for your time. Thanks again.
-
Runium about 11 years@EvanTeitelman: Thanks a lot, but no need for bounty points. Keep your hard earned points for a rainy day ;). When it comes to GPT this read might interest you.