Having View Slide in From Bottom in Swift?
14,959
Solution 1
Swift 5 version:
import UIKit
class ViewController: UIViewController {
let collectionView: UICollectionView = {
let frame = CGRect(x: 0, y: 50, width: UIScreen.main.bounds.size.width, height: UIScreen.main.bounds.size.height - 50)
let col = UICollectionView(frame: frame, collectionViewLayout: UICollectionViewFlowLayout())
col.layer.borderColor = UIColor.red.cgColor
col.layer.borderWidth = 1.0
col.backgroundColor = UIColor.yellow
return col
}()
let switchView = UISwitch()
func switched(s: UISwitch){
let origin: CGFloat = s.on ? view.frame.height : 50
UIView.animate(withDuration: 0.35) {
self.collectionView.frame.origin.y = origin
}
}
override func viewDidLoad() {
super.viewDidLoad()
switchView.frame = CGRect(x: 0, y: 20, width: 40, height: 20)
switchView.addTarget(self, action: #selector(switched), forControlEvents: .valueChanged)
view.addSubview(switchView)
view.addSubview(collectionView)
}
}
I've made a little demo for you: created new project and wrote that code in ViewController.swift, nothing more. Think it helps
import UIKit
class ViewController: UIViewController {
let collectionView: UICollectionView = {
let frame = CGRect(x: 0, y: 50, width: UIScreen.mainScreen().bounds.size.width, height: UIScreen.mainScreen().bounds.size.height - 50)
let col = UICollectionView(frame: frame, collectionViewLayout: UICollectionViewFlowLayout())
col.layer.borderColor = UIColor.redColor().CGColor
col.layer.borderWidth = 1.0
col.backgroundColor = UIColor.yellowColor()
return col
}()
let switchView = UISwitch()
func switched(s: UISwitch){
let origin: CGFloat = s.on ? view.frame.height : 50
UIView.animateWithDuration(0.35) {
self.collectionView.frame.origin.y = origin
}
}
override func viewDidLoad() {
super.viewDidLoad()
switchView.frame = CGRect(x: 0, y: 20, width: 40, height: 20)
switchView.addTarget(self, action: #selector(switched), forControlEvents: .ValueChanged)
view.addSubview(switchView)
view.addSubview(collectionView)
}
}
Solution 2
SwiftStudier's answer updated for Swift 3:
import UIKit
class ViewController: UIViewController {
let collectionView: UICollectionView = {
let frame = CGRect(x: 0, y: 50, width: UIScreen.main.bounds.size.width, height: UIScreen.main.bounds.size.height - 50)
let col = UICollectionView(frame: frame, collectionViewLayout: UICollectionViewFlowLayout())
col.layer.borderColor = UIColor.red.cgColor
col.layer.borderWidth = 1.0
col.backgroundColor = UIColor.yellow
return col
}()
let switchView = UISwitch()
func switched(s: UISwitch){
let origin: CGFloat = s.isOn ? view.frame.height : 50
UIView.animate(withDuration: 0.35) {
self.collectionView.frame.origin.y = origin
}
}
override func viewDidLoad() {
super.viewDidLoad()
switchView.frame = CGRect(x: 0, y: 20, width: 40, height: 20)
switchView.addTarget(self, action: #selector(switched), for: .valueChanged)
view.addSubview(switchView)
view.addSubview(collectionView)
}
}
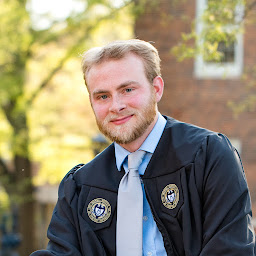
Author by
Mike Schmidt
Updated on June 05, 2022Comments
-
Mike Schmidt almost 2 years
If I have a view setup in my storyboard, is there a way that I can have that view (of a custom width and height) slide up from the bottom of my screen when a button is pressed? I would want the screen to just overlay (so that you would still be able to press things on the screen underneath).
How would I set this up?
func isChecked(){ let window = UIApplication.sharedApplication().keyWindow window!.addSubview(collectionView) let height: CGFloat = 250 let y = window!.frame.height - 250 collectionView.frame = CGRect(x: 0, y: window!.frame.height, width: window!.frame.width, height: height) UIView.animateWithDuration(0.5, delay: 0, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .CurveEaseOut, animations: { self.collectionView.frame = CGRectMake(0, y, self.collectionView.frame.width, self.collectionView.frame.height) }, completion: nil) } func isUnchecked(){ let window = UIApplication.sharedApplication().keyWindow UIView.animateWithDuration(0.3, animations: { self.collectionView.frame = CGRectMake(0, window!.frame.height, self.collectionView.frame.width, self.collectionView.frame.height) }) }
Is there a way that I can accomplish what I am doing above, except with a view that I create in my storyboard?