Hot reload on save
Solution 1
Sorry for the plug, but I wrote a very simple plugin to handle this.
It makes use of Flutter's --pid-file
command line flag to send it a SIGUSR1
signal.
You can achieve the same result as my two-line plugin by adding this to an autocmd
silent execute '!kill -SIGUSR1 "$(cat /tmp/flutter.pid)"'
And launching Flutter with the --pid-file
flag.
Solution 2
I made a vim plugin hankchiutw/flutter-reload.vim based on killing with SIGUSR1
.
You don't have to use --pid-file
flag with this plugin. (Thanks to the pgrep
:))
Simply execute flutter run
, modify your *.dart file and see the reloading.
Solution 3
I did it with the excellent little tool called entr
. On OS/X you can install it from brew
: brew install entr
. The home page of the tool is at http://eradman.com/entrproject/
Then you start flutter run
with the pidfile as @nobody_nowhere suggests.
How do you run entr
depends on the level of service. In the simplest case you just do find lib/ -name '*.dart' | entr -p kill -USR1 $(cat /tmp/flutter.pid)
But such invocation will not detect new files in the source tree (because find
builds a list of files to watch only once, at the start). You can get away with slightly more complex one-liner:
while true
do
find lib/ -name '*.dart' | \
entr -d -p kill -USR1 $(cat /tmp/flutter.pid)
done
The -d
option makes entr
exit when it does detect a new file in one of the directories and the loop runs again.
I personally use even more complex approach. I use Redux and change to middleware or other state files does not work with hot reload, it doesn't pick up these changes. So you need to resort to hot restart.
I have a script hotrestarter.sh
:
#!/bin/bash
set -euo pipefail
PIDFILE="/tmp/flutter.pid"
if [[ "${1-}" != "" && -e $PIDFILE ]]; then
if [[ "$1" =~ \/state\/ ]]; then
kill -USR2 $(cat $PIDFILE)
else
kill -USR1 $(cat $PIDFILE)
fi
fi
It checks if the modified file lives in /state
subdirectory and if true does hot restart or else hot reload. I call the script like that:
while true
do
find lib/ -name '*.dart' | entr -d -p ./hotreloader.sh /_
done
The /_
parameter makes entr
to pass the name of the file to the program being invoked.
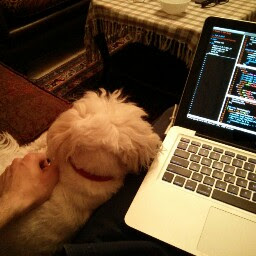
Bashar
Updated on December 04, 2022Comments
-
Bashar over 1 year
I'm currently using a terminal and vim on OSX as a development environment for Flutter. Things are going pretty well except that the app does not reload when I save any dart files. Is there a way to trigger that behavior?Currently I have to go to the terminal and hit "r" to see my changes.