How can I accept the backspace key in the keypress event?
Solution 1
I like to use !
Char.IsControl(e.KeyChar) so that all the "control" characters like the backspace key and clipboard keyboard shortcuts are exempted.
If you just want to check for backspace, you can probably get away with:
if (e.KeyChar == (char)8 && ...)
Solution 2
I use the two following segments alot:
This one for restricting a textbox to integer only, but allowing control keys:
if (Char.IsDigit(e.KeyChar)) return;
if (Char.IsControl(e.KeyChar)) return;
e.Handled = true;
This one for restricing a textbox to doubles, allowing one '.' only, and allowing control keys:
if (Char.IsDigit(e.KeyChar)) return;
if (Char.IsControl(e.KeyChar)) return;
if ((e.KeyChar == '.') && ((sender as TextBox).Text.Contains('.') == false)) return;
if ((e.KeyChar == '.') && ((sender as TextBox).SelectionLength == (sender as TextBox).TextLength)) return;
e.Handled = true;
Solution 3
You have to add !(char.IsControl(e.KeyChar)) in you sentence and that's it.
private void txtNombre_KeyPress(object sender, KeyPressEventArgs e)
{
if (!(char.IsLetter(e.KeyChar)) && !(char.IsNumber(e.KeyChar)) && !(char.IsControl(e.KeyChar)) && !(char.IsWhiteSpace(e.KeyChar)))
{
e.Handled = true;
}
}
Solution 4
The backspace key does not raised by KeyPress event. So you need to catch it in KeyDown or KeyUp events and set SuppressKeyPress property is true to prevent backspace key change your text in textbox:
private void txtAdd_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Back)
{
e.SuppressKeyPress = true;
}
}
Solution 5
for your problem try this its work for when backspace key pressed
e.KeyChar == ((char)Keys.Back)
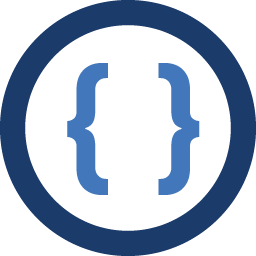
Admin
Updated on March 26, 2021Comments
-
Admin over 3 years
This is my code:
private void txtAdd_KeyPress(object sender, KeyPressEventArgs e) { if (!(char.IsLetter(e.KeyChar)) && !(char.IsNumber(e.KeyChar)) && !(char.IsWhiteSpace(e.KeyChar))) { e.Handled = true; } }
It allows me to enter letters, numbers and spaces but it doesn't allow me to do backspace. Please help me.