How can I access an array declared in an HTML hidden input from a Javascript function?
Solution 1
Naming a hidden input sq[]
doesn't change it into an array. It's still just a text field essentially. You will need to access the field and parse the value as a comma-separated list (or JSON or whatever format you choose).
Assuming you have a form like so:
<form name="form1">
<input type="hidden" name="sq[]" value="a,b,c,d" />
<input type="hidden" name="sqjson[]" value='["e","f","g","h"]' />
</form>
You can access the values using split [MDN]:
var arr = document.form1['sq[]'].value.split(',');
for (var ii = 0; ii < arr.length; ii++) {
console.log(arr[ii]);
}
Or using JSON.parse [MDN], which would make more complex objects easier to store in a hidden field:
var arr = JSON.parse(document.form1['sqjson[]'].value);
for (var ii = 0; ii < arr.length; ii++) {
console.log(arr[ii]);
}
Click here for a working version of this example.
Solution 2
You can store sq
array in JSON format in the hidden field.
<input type="hidden" name="sq" value="[]" ></input>
Then using JSON.parse
and JSON.stringify
you can deserialize from string value to memory object, add/remove values in the array, then storing it back to the hidden field.
var $sq = JSON.parse($("#sq").val());
...
$("#sq").val(JSON.stringify($sq);
Without JQuery:
var hf = document.getElementById("sq");
var sq = JSON.parse(hf.value);
...
hf.value = JSON.stringify(sq);
Then you can pass sq
as parameter to functions where needed. Or store it in global variable.
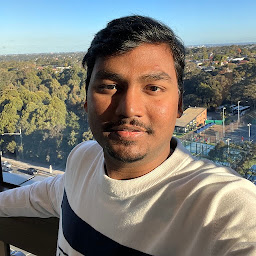
PRASHANT KUMAR
I am a Web Developer, hungry to learn new technologies, languages. Knows back-end and front-end development. In the field of web development for me it take hardly 2-3 days to learn new technology and start working on that. Current skills are in C, Java, Servlet, JSP, Mysql, HTML/HTML5, CSS/CSS3, Bootstrap 3 Javascript, Jquery, AngularJs, salesforce-lightning with aura framework.
Updated on June 14, 2022Comments
-
PRASHANT KUMAR about 2 years
I have declared an array in a hidden input in my HTML form. Now I want to access that array in a Javascript function. For this, I have written the following code:
<form name="form1"> <input type="hidden" name="sq[]" ></input> <input type="hidden" name="a" ></input> </form>
And in the Javascript function:
function myfunction() { document.form1.a.value=i; // Here, I can access the variable 'a' // (since a is not in the form of array) var i; for(i=0;i<4;i++) { document.form1.sq[i].value=i; // Here, I am not able to access array named sq[]. } }
-
FishBasketGordo almost 10 yearsThis solution requires jQuery, which the OP does not appear to be using.
-
PRASHANT KUMAR almost 10 years@Francois B. i want to first initialize the array and than use this to modify the webpage(by using document.getElementById("id_of_div").innerHTML=sq[i]) in another function.