How can I automatically convert PowerPoint to PDF?
Solution 1
No third party product is needed. As you've noted, PowerPoint can export a presentation as a PDF. With the application of a little scripting, you can achieve your result. I've whipped up the VB Script below. Simply create a file with a name ending in ".vbs", paste the code below.
To use:
CSCRIPT ppt.vbs "input file name" "output file name"
It's important to note:
- If the name(s) contain spaces, they'll need to be quoted.
- If you don't specify a path for the output file, PowerPoint will put it in your Documents folder.
I've included links inline for references to the various bits.
Option Explicit
Sub WriteLine ( strLine )
WScript.Stdout.WriteLine strLine
End Sub
' http://msdn.microsoft.com/en-us/library/office/aa432714(v=office.12).aspx
Const msoFalse = 0 ' False.
Const msoTrue = -1 ' True.
' http://msdn.microsoft.com/en-us/library/office/bb265636(v=office.12).aspx
Const ppFixedFormatIntentScreen = 1 ' Intent is to view exported file on screen.
Const ppFixedFormatIntentPrint = 2 ' Intent is to print exported file.
' http://msdn.microsoft.com/en-us/library/office/ff746754.aspx
Const ppFixedFormatTypeXPS = 1 ' XPS format
Const ppFixedFormatTypePDF = 2 ' PDF format
' http://msdn.microsoft.com/en-us/library/office/ff744564.aspx
Const ppPrintHandoutVerticalFirst = 1 ' Slides are ordered vertically, with the first slide in the upper-left corner and the second slide below it.
Const ppPrintHandoutHorizontalFirst = 2 ' Slides are ordered horizontally, with the first slide in the upper-left corner and the second slide to the right of it.
' http://msdn.microsoft.com/en-us/library/office/ff744185.aspx
Const ppPrintOutputSlides = 1 ' Slides
Const ppPrintOutputTwoSlideHandouts = 2 ' Two Slide Handouts
Const ppPrintOutputThreeSlideHandouts = 3 ' Three Slide Handouts
Const ppPrintOutputSixSlideHandouts = 4 ' Six Slide Handouts
Const ppPrintOutputNotesPages = 5 ' Notes Pages
Const ppPrintOutputOutline = 6 ' Outline
Const ppPrintOutputBuildSlides = 7 ' Build Slides
Const ppPrintOutputFourSlideHandouts = 8 ' Four Slide Handouts
Const ppPrintOutputNineSlideHandouts = 9 ' Nine Slide Handouts
Const ppPrintOutputOneSlideHandouts = 10 ' Single Slide Handouts
' http://msdn.microsoft.com/en-us/library/office/ff745585.aspx
Const ppPrintAll = 1 ' Print all slides in the presentation.
Const ppPrintSelection = 2 ' Print a selection of slides.
Const ppPrintCurrent = 3 ' Print the current slide from the presentation.
Const ppPrintSlideRange = 4 ' Print a range of slides.
Const ppPrintNamedSlideShow = 5 ' Print a named slideshow.
' http://msdn.microsoft.com/en-us/library/office/ff744228.aspx
Const ppShowAll = 1 ' Show all.
Const ppShowNamedSlideShow = 3 ' Show named slideshow.
Const ppShowSlideRange = 2 ' Show slide range.
'
' This is the actual script
'
Dim inputFile
Dim outputFile
Dim objPPT
Dim objPresentation
Dim objPrintOptions
Dim objFso
If WScript.Arguments.Count <> 2 Then
WriteLine "You need to specify input and output files."
WScript.Quit
End If
inputFile = WScript.Arguments(0)
outputFile = WScript.Arguments(1)
Set objFso = CreateObject("Scripting.FileSystemObject")
If Not objFso.FileExists( inputFile ) Then
WriteLine "Unable to find your input file " & inputFile
WScript.Quit
End If
If objFso.FileExists( outputFile ) Then
WriteLine "Your output file (' & outputFile & ') already exists!"
WScript.Quit
End If
WriteLine "Input File: " & inputFile
WriteLine "Output File: " & outputFile
Set objPPT = CreateObject( "PowerPoint.Application" )
objPPT.Visible = True
objPPT.Presentations.Open inputFile
Set objPresentation = objPPT.ActivePresentation
Set objPrintOptions = objPresentation.PrintOptions
objPrintOptions.Ranges.Add 1,objPresentation.Slides.Count
objPrintOptions.RangeType = ppShowAll
' Reference for this at http://msdn.microsoft.com/en-us/library/office/ff746080.aspx
objPresentation.ExportAsFixedFormat outputFile, ppFixedFormatTypePDF, ppFixedFormatIntentScreen, msoTrue, ppPrintHandoutHorizontalFirst, ppPrintOutputSlides, msoFalse, objPrintOptions.Ranges(1), ppPrintAll, "Slideshow Name", False, False, False, False, False
objPresentation.Close
ObjPPT.Quit
Solution 2
You can print to a PDF printer driver like Adobe Distiller, or any of the many cheaper or even opensource drivers out there.
Solution 3
Office PowerPoint Viewer 2007 has a command line switch /p
which will let you print a PowerPoint file to a default printer.
For example:
Send the presentation to a printer, and print the file.
Example: "c:\program files\microsoft office\office12\PPTVIEW.exe" /P "Presentation.pptx"
This example prints the Presentation.pptx file.
The PDF Printer would likely have to set as your default printer.
Rather than Adobe Distiller which means you have to buy Adobe Acrobat, I recommend you use PDFCreator. It is free and lets you save the output file in an automated way if you tweak the options. This way, you can have a completely command line method to convert PowerPoint files to PDF without having to make additional payments to Microsoft nor Adobe.
Solution 4
Used this to write a script for converting a whole folder, please reply if this can be improved this is my first time writing vbscript!
command:
cscript scriptname.vbs "C:/path/to/folder"
files will be saved in the directory the script is in.
code:
Option Explicit
Sub WriteLine ( strLine )
WScript.Stdout.WriteLine strLine
End Sub
Const msoFalse = 0 ' False.
Const msoTrue = -1 ' True.
Const ppFixedFormatIntentScreen = 1 ' Intent is to view exported file on screen.
Const ppFixedFormatIntentPrint = 2 ' Intent is to print exported file.
Const ppFixedFormatTypeXPS = 1 ' XPS format
Const ppFixedFormatTypePDF = 2 ' PDF format
Const ppPrintHandoutVerticalFirst = 1 ' Slides are ordered vertically, with the first slide in the upper-left corner and the second slide below it.
Const ppPrintHandoutHorizontalFirst = 2 ' Slides are ordered horizontally, with the first slide in the upper-left corner and the second slide to the right of it.
Const ppPrintOutputSlides = 1 ' Slides
Const ppPrintOutputTwoSlideHandouts = 2 ' Two Slide Handouts
Const ppPrintOutputThreeSlideHandouts = 3 ' Three Slide Handouts
Const ppPrintOutputSixSlideHandouts = 4 ' Six Slide Handouts
Const ppPrintOutputNotesPages = 5 ' Notes Pages
Const ppPrintOutputOutline = 6 ' Outline
Const ppPrintOutputBuildSlides = 7 ' Build Slides
Const ppPrintOutputFourSlideHandouts = 8 ' Four Slide Handouts
Const ppPrintOutputNineSlideHandouts = 9 ' Nine Slide Handouts
Const ppPrintOutputOneSlideHandouts = 10 ' Single Slide Handouts
Const ppPrintAll = 1 ' Print all slides in the presentation.
Const ppPrintSelection = 2 ' Print a selection of slides.
Const ppPrintCurrent = 3 ' Print the current slide from the presentation.
Const ppPrintSlideRange = 4 ' Print a range of slides.
Const ppPrintNamedSlideShow = 5 ' Print a named slideshow.
Const ppShowAll = 1 ' Show all.
Const ppShowNamedSlideShow = 3 ' Show named slideshow.
Const ppShowSlideRange = 2 ' Show slide range.
'
' This is the actual script
'
Dim inputDirectory
Dim inputFolder
Dim inFiles
Dim outputFolder
Dim inputFile
Dim outputFile
Dim curFile
Dim objPPT
Dim objPresentation
Dim objPrintOptions
Dim objFso
Dim curDir
If WScript.Arguments.Count <> 1 Then
WriteLine "You need to specify input files."
WScript.Quit
End If
Set objFso = CreateObject("Scripting.FileSystemObject")
curDir = objFso.GetAbsolutePathName(".")
Set inputFolder = objFSO.GetFolder(WScript.Arguments.Item(0))
Set outputFolder = objFSO.GetFolder(WScript.Arguments.Item(0))
Set inFiles = inputFolder.Files
Set objPPT = CreateObject( "PowerPoint.Application" )
For Each curFile in inFiles
Set inputFile = curFile
If Not objFso.FileExists( inputFile ) Then
WriteLine "Unable to find your input file " & inputFile
WScript.Quit
End If
objPPT.Visible = TRUE
objPPT.Presentations.Open inputFile
Set objPresentation = objPPT.ActivePresentation
Set objPrintOptions = objPresentation.PrintOptions
objPrintOptions.Ranges.Add 1,objPresentation.Slides.Count
objPrintOptions.RangeType = ppShowAll
objPresentation.ExportAsFixedFormat curDir & curFile.Name & ".pdf", ppFixedFormatTypePDF, ppFixedFormatIntentScreen, msoTrue, ppPrintHandoutHorizontalFirst, ppPrintOutputSlides, msoFalse, objPrintOptions.Ranges(1), ppPrintAll, "Slideshow Name", False, False, False, False, False
objPresentation.Close
Next
ObjPPT.Quit
Related videos on Youtube
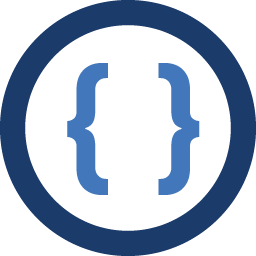
Admin
Updated on September 18, 2022Comments
-
Admin over 1 year
I need to convert .ppt/.pptx files to .pdf files (or images) through the command line using a 3rd party product.
I'm using this for a Windows 2008 server and I can't use any GUI or website as this needs to be an automated process.
I've tried libreoffice, but it has issues converting smart art.
EDIT: My final solution was to use the powerpoint interop with C#. See also: https://stackoverflow.com/questions/26372020/how-to-programmatically-create-a-powerpoint-from-a-list-of-images
-
strangetimes over 10 yearsThe Adobe Distiller official page says nothing about converting powerpoint files to .pdf's.
-
Rod MacPherson over 10 yearsIt is a print driver, anything you can print you can make into a PDF.
-
Sun over 10 yearsHow do you actually run PowerPoint to print to the Adobe Distiller print driver in an automated way?
-
peter.ruzicska over 10 yearsI tried this, but it opens up a GUI dialog box instead of silently following orders. Am I doing it wrong?
-
Sun over 10 yearsMaybe /p only brings you up to the print dialog as the default printer is not selected and processed. You can try /pt see if the PowerPoint equivalent works for the PowerPoint Viewer. This would be a workaround, but you could also create an AutoHotKey macro to click on Print for you when you get to the GUI prompt. Other you can buy PowerPoint and use the /pt parameter which I think works better in your case.
-
peter.ruzicska over 10 yearsI tried using the
/pt
option for PowerPoint, but nothing happens. Probably because it treats the pdfCreator printer as an actual physical printer. It's not printing to a file, which is what I want. -
Rod MacPherson over 10 yearsuse the /pt option from the command line to print and specify which printer. officeone.mvps.org/pptfaq/ppt_cmdline.html Setting document defaults in distiller should get you past the print options GUI pop-up.
-
strangetimes over 10 years@RodMacPherson I tried this in the comment section of another answer. It didn't work. When I tried to print it using a pdf printer driver, it just gave me a non-pdf file
-
peter.ruzicska over 10 yearsI know that PowerPoint has the option to simply save it as a .pdf, which is what I want, but I can't do that with the command line
-
Rod MacPherson over 10 years@OWiz Since you have PDFCreator already let's focus on that. I don't intend to recommend one driver over another, so let's go with what you have. Try to print something to PDF creator in GUI mode. Once it pops up go into the Options menu, and enable autosave. Save your settings and give it a try from the command line.
-
strangetimes over 10 years@RodMacPherson I can save the files with PDFCreator using the GUI, but it has the same formatting problem that libreoffice does.
-
Sun over 10 yearsYou may also want to explore the /M parameter which would run a macro. That macro can call a VBA script which would save your PowerPoint to a PDF using the built-in PowerPoint functions. Here is an example: vbadud.blogspot.com/2012/05/…
-
Admin over 10 yearsThank you. I was just about to write something like this, except in C#. The only downside, is that the Powerpoint window pops up for a second or two. I tried setting
objPPT.Visible
toFalse
, but it errored and told me that it wasn't allowed. -
Admin over 10 yearsThis process is going to be done on a server. I imagine that it would be pretty resource-consuming to actually open up PowerPoint every single time a file has to be converted. Is there a way to not do that?
-
strangetimes over 10 years@RodMacPherson I came back to the PDFCreator solution later, and it worked, however, I found it impossible to programmatically run the console commands from C#.
-
Tripartio over 7 yearsIf your code is a modification of BillP3rd's answer, then it would be good to cite it properly (see chitu.okoli.org/psyche/it/how-to-cite-code). Also, I'm not sure why you deleted the comments where BillP3rd cited his own sources.
-
Tripartio over 7 yearsMostly works, though it remained the files with the folder name in my case ( I used "." as the input folder).
-
iBug over 5 years@Houseman You can avoid launching and closing PowerPoint repeatedly by modifying the script, but every single file needs to be opened and closed.
-
Marcel over 5 yearsIs it possible to adapt this to work with word?
-
bers over 3 yearsThis works great. For some reason, though, this solution has the
FrameSlides
parameter set tomsoTrue
(this one:objPresentation.ExportAsFixedFormat ... ppFixedFormatIntentScreen, msoTrue
). Set tomsoFalse
to avoid having a black frame around your exported pdf. -
bers over 3 years@Marcel see gist.github.com/Iambecomeroot/395f802a094e433c25a253577988f09d for example.
-
bers over 3 yearsYou may want to fix quotation marks in this line:
WriteLine "Your output file (' & outputFile & ') already exists!"
-
Borislav Markov almost 3 yearsThank you, you are great
-
Tompa over 2 yearsThanks, I find that I need absolute path for the input file (maybe output file as well), else I got "The system cannot find the file specified." by the line objPPT.Presentations.Open inputFile, using a relative path.