How can I call a sqlserver function from VB.net(or C#) ? Is there some syntax like stored procedure?
37,437
Solution 1
Sorry, there is no way to run a function directly. Either call it using a sql Text command
Public Sub RunFunction(ByVal input As Short)
Using myConnection As New Data.SqlClient.SqlConnection
Using myCommand As New Data.SqlClient.SqlCommand("Select dbo.MyFunction(@MyParam)", myConnection)
myCommand.CommandType = CommandType.Text
myCommand.Parameters.Add(New Data.SqlClient.SqlParameter("@MyParam", input))
myCommand.CommandTimeout = 0
Try
myCommand.ExecuteNonQuery()
Catch ex As Exception
End Try
End Using
End Using
End Sub
Or Wrap a procedure round it...
Create Procedure RunMyFunction(@MyParam as int)
Select * FROM dbo.MyFunction(@MyParam)
Go
Solution 2
Yes you can call a function directly as demonstrated below.
Dim dtaName As New SqlClient.SqlDataAdapter
dtaName.SelectCommand = New SqlClient.SqlCommand
With dtaName.SelectCommand
.CommandTimeout = 60
.Connection = prvcmpINC.cntINC
.CommandType = CommandType.StoredProcedure
.CommandText = "dbo.app_GetName"
.Parameters.AddWithValue("@ParamToPassIn", parstrParamToPassIn)
.Parameters.Add("@intResult", SqlDbType.Int)
.Parameters("@intResult").Direction = ParameterDirection.ReturnValue
End With
dtaName.SelectCommand.ExecuteScalar()
intRuleNo = dtaName.SelectCommand.Parameters("@intResult").Value
Solution 3
This works for me and is based on one of the above answers using a SqlDataAdapter
(note that you do not need to use one) and ExecuteScalar
(can use ExecuteNonQuery
as shown here):
bool res = false;
using (SqlConnection conn = new SqlConnection(GetConnectionString()))
{
using (SqlCommand comm = new SqlCommand("dbo.MyFunction", conn))
{
comm.CommandType = CommandType.StoredProcedure;
SqlParameter p1 = new SqlParameter("@MyParam", SqlDbType.Int);
// You can call the return value parameter anything, .e.g. "@Result".
SqlParameter p2 = new SqlParameter("@Result", SqlDbType.Bit);
p1.Direction = ParameterDirection.Input;
p2.Direction = ParameterDirection.ReturnValue;
p1.Value = myParamVal;
comm.Parameters.Add(p1);
comm.Parameters.Add(p2);
conn.Open();
comm.ExecuteNonQuery();
if (p2.Value != DBNull.Value)
res = (bool)p2.Value;
}
}
return res;
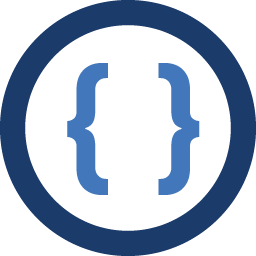
Author by
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Public Sub cleanTables(ByVal prOKDel As Short) Dim sqlParams(1) As SqlParameter Dim sqlProcName As String sqlProcName = "db.dbo.sp_mySP" sqlParams(1) = New SqlParameter("@OKDel", prOKDel) Try dbConn.SetCommandTimeOut(0) dbConn.ExecuteNonQuery(CommandType.StoredProcedure, sqlProcName, sqlParams) Catch ex As Exception Finally End Try End Sub
Is there
CommandType.StoredProcedure...CommandType.Function sqlParams(1) = New SqlParameter("@OKDel", prOKDel)...
and finally datatable dt = dbConn.Execute(CommandType.StoredProcedure, sqlProcName, sqlParams)
Thanks