how can i call web api Controller from ajax
21,665
Basically it's a case sensitivity problem on URL
vs url
combined with some unnecessary work. So change your script to:
<script type="text/javascript">
function Login() {
var Login = {};
Login.username = $("#txtUserName").val();
Login.password = $("#txtPassword").val();
$.ajax({
url: '/api/Login/',
method: 'POST',
dataType: 'json',
contentType: 'application/json; charset=utf-8',
data: Login,
success: function (data) {
alert("Saved successfully");
},
fail : function( jqXHR, textStatus ) {
alert( "Request failed: " + textStatus );
}
})
}
</script>
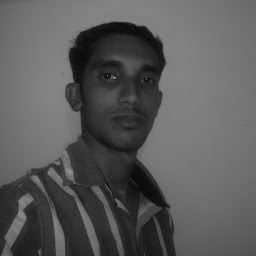
Author by
Ranjith prasad
Updated on March 06, 2020Comments
-
Ranjith prasad about 4 years
I am a beginner of ASP MVC with web api.
By using below codes I tried to call a function which is written at the controller. For checking I used breakpoint so the control could not go to the controller so that I can not track actually what is going on.
The given code explains how to pass username and password to the controller:
<script type="text/javascript"> function Login() { var Login = {}; Login.username = document.getElementById("txtUserName").value; Login.password = document.getElementById("txtPassword").value; $.ajax({ URL: 'api/Login/' + Login, type: 'POST', dataType: 'json', contentType: 'application/json; charset=utf-8', data: JSON.stringify(Login), success: function (data) { alert("Saved successfully"); } }) } </script>
This is the controller which i used to pass
public class LoginController : ApiController { [HttpPost] public void Login(Login login) { string user = login.UserName.ToString(); string password = login.Password.ToString(); } }