How can I change color of part of the text in QLineEdit?
14,823
Solution 1
Just found a neat trick for that.
static void setLineEditTextFormat(QLineEdit* lineEdit, const QList<QTextLayout::FormatRange>& formats)
{
if(!lineEdit)
return;
QList<QInputMethodEvent::Attribute> attributes;
foreach(const QTextLayout::FormatRange& fr, formats)
{
QInputMethodEvent::AttributeType type = QInputMethodEvent::TextFormat;
int start = fr.start - lineEdit->cursorPosition();
int length = fr.length;
QVariant value = fr.format;
attributes.append(QInputMethodEvent::Attribute(type, start, length, value));
}
QInputMethodEvent event(QString(), attributes);
QCoreApplication::sendEvent(lineEdit, &event);
}
static void clearLineEditTextFormat(QLineEdit* lineEdit)
{
setLineEditTextFormat(lineEdit, QList<QTextLayout::FormatRange>());
}
// Usage example:
QLineEdit* lineEdit = new QLineEdit;
lineEdit->setText(tr("Task Tracker - Entry"));
QList<QTextLayout::FormatRange> formats;
QTextCharFormat f;
f.setFontWeight(QFont::Bold);
QTextLayout::FormatRange fr_task;
fr_task.start = 0;
fr_task.length = 4;
fr_task.format = f;
f.setFontItalic(true);
f.setBackground(Qt::darkYellow);
f.setForeground(Qt::white);
QTextLayout::FormatRange fr_tracker;
fr_tracker.start = 5;
fr_tracker.length = 7;
fr_tracker.format = f;
formats.append(fr_task);
formats.append(fr_tracker);
setLineEditTextFormat(lineEdit, formats);
Solution 2
You can change the color with the use of style sheets.
QLineEdit* myLineEdit = new QLineEdit("Whatever");
//for whatever case you want to change the color
if(syntax_needs_to_highlighted)
myLineEdit->setStyleSheet("QLineEdit#myLineEdit{color:blue}");
You may want to consider using QTextBrowser
for this case.
Solution 3
You can change color of texts like this:
QLineEdit *line = new QLineEdit();
line->setText("this is a test");
line->setStyleSheet("foreground-color: blue;");
If it won't work, replace the last line with the following:
line->setStyleSheet("color: blue;");
Related videos on Youtube
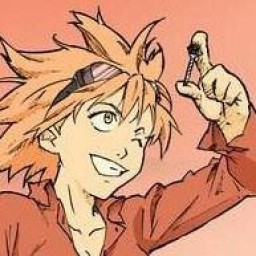
Comments
-
Vasaka 8 months
I want to add some syntax highlighting to text being written in QLineEdit, but it does not support rich text formatting, I can not change QlineEdit to something else, so I should find how to set color of text in this widget.
Is there a way to do this?
-
Tom Myddeltyn almost 7 yearsneither worked needed to use
"color: blue;"
(FWIW using PySide) -
Petross404 about 1 yearBut the tag is about c++, not python.
-
SixenseMan about 1 yearwell it's still Qt... just do the same thing in c++