How can I create columns with type Date and type DateTime in nestjs with typeORM?
51,656
Solution 1
You can see the docs here that explains the @Column decorator. In @Column there is an option called type -> here is where you specify which type of date you want to store for that specific column.
More on column types here.
For example (using PostgreSQL):
@Column({ type: 'date' })
date_only: string;
@Column({ type: 'timestamptz' }) // Recommended
date_time_with_timezone: Date;
@Column({ type: 'timestamp' }) // Not recommended
date_time_without_timezone: Date;
Note that date_only
is of type string. See this issue for more information.
Hope it helps :)
Solution 2
How about ?
@CreateDateColumn()
created_at: Date;
@UpdateDateColumn()
updated_at: Date;
EDIT
You can find more info here
Solution 3
To add more to this ticket as DateTime was not even mentioned:
/**
* Start DateTime
*/
@Column({
type: 'datetime',
default: () => 'NOW()',
})
@Index()
start: string;
/**
* End DateTime
*/
@Column({
type: 'datetime',
nullable: true,
})
@Index()
end: string;
This is for those not wanting or needing to use
@CreateDateColumn()
@UpdateDateColumn()
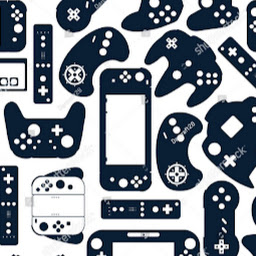
Author by
kri-dev
Updated on February 09, 2022Comments
-
kri-dev over 2 years
I am new with nestjs. How can I set columns that accepts Date format and dateTime format?
Not in both cases, the columns are two differents column, one accept Date and other dateTime.
-
sarfata about 3 yearsThis answer is not correct. The type returned by typeorm for
type: 'date'
is a string - not a date object with the time set to 0. See github.com/typeorm/typeorm/issues/2176 -
Carlo Corradini about 3 years@sarfata I've updated my answer. Let me know what you think!
-
sarfata about 3 years@CarloCorradini awesome! I like that you clearly recommend
timestamptz
too ;) -
Jeremy over 2 yearswould timestamp be ok when using mysql? as timestamptz is not supported?
-
thefern about 2 yearsThis should be the correct answer, simple and handled automatically by typeorm on save.
-
Eazash almost 2 yearsI don't believe this should be the correct answer, or upvoted as it is. There are more uses for timestamp columns than just created_at and updated_at values
-
Luis Contreras almost 2 years@Eazash that's why I provided a link to the rest of the docs :D
-
Eugene Zalivadnyi almost 2 yearsOk, but how to use "timestamptz" with GraphQL? What should I use inside ObjectType and InputType to fix the issue with the wrong DateTime types?
-
Carlo Corradini almost 2 years@EugeneZalivadnyi I use GraphQL Scalars library with a date/time scalar mapping. E.g.
GraphQLTimestamp
for UNIX epoch orGraphQLDateTime
for UTC. You decide what best suits your needs since the input given to the scalar is always aDate
instance (timestamptz
).