How can I determine a file's creation date in Windows?
Solution 1
Use stat function
see here
#include <sys/stat.h>
#include <unistd.h>
#include <time.h>
struct tm* clock; // create a time structure
struct stat attrib; // create a file attribute structure
stat("afile.txt", &attrib); // get the attributes of afile.txt
clock = gmtime(&(attrib.st_mtime)); // Get the last modified time and put it into the time structure
Solution 2
On Windows, you should use the GetFileAttributesEx function for that.
Solution 3
For C, it depends on which operating system you are coding for. Files are a system dependent concept.
If you're system has it, you can go with the stat()
(and friends) function: http://pubs.opengroup.org/onlinepubs/009695399/functions/stat.html.
On Windows you may use the GetFileTime()
function: http://msdn.microsoft.com/en-us/library/ms724320%28v=vs.85%29.aspx.
Solution 4
Unix systems don't store the time of file creation. Unix systems do store the last time the file was read (if atime
is turned on for that specific file system; sometimes it is disabled for speed), the last time the file was modified (mtime
), and the last time the file's metadata changed (ctime
).
See the stat(2)
manpage for details on using it.
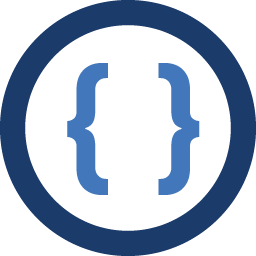
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
How can I get the date of when a file was created? I am running Windows.
-
Cody Gray over 13 yearsDoes this work on Windows? Or are you assuming the OP is using UNIX?
-
Arsen Mkrtchyan over 13 yearsYea, I guess , see msdn.microsoft.com/en-us/library/14h5k7ff%28v=vs.80%29.aspx
-
Billy ONeal over 13 yearsErr.. I see no
stat
in that link. I do see a_stat
. -
Billy ONeal over 13 years
GetFileAttributesEx
is superior toGetFileTime
for this particular purpose, for three reasons: 1. UsingGetFileTime
requires opening a handle to the file (and therefore the handle must be managed correctly) 2. As a result of (1), performance can suffer because the system actually has to seek to the file rather than it's MFT position 3. As a result of (1),GetFileAttributesEx
usually requires fewer permissions thanGetFileTime
, and automatically asks for the minimal permissions require to retrieve the file's metadata rather than the file itself. -
Alexander Leon VI over 8 yearsI am using this approach be cause I want to know the creation date of several files, but I get the same value for all the files in the
ftCreationTime.dwLowDateTime
and ` ftCreationTime.dwLowDateTime `, why is happen this? Thank's -
Billy ONeal over 8 years@Alexander: Perhaps the files have the same value for all of them?
-
Alexander Leon VI over 8 yearsThe files have the same date, but I was expecting some differences due to all of them have different hour