How can I display terminal output to a text box in PyQt
10,680
Try it:
from PyQt5 import QtCore, QtGui, QtWidgets
import numpy as np
#from Distances import radial_distance
def radial_distance(body1, body2, utc, ref, abcorr, obs):
x_1 = 1
x_2 = 4
y_1 = 5
y_2 = 2
z_1 = 7
z_2 = 6
d_rad = np.sqrt((x_2 - x_1)**2.0 + (y_2 - y_1)**2.0 + (z_2 - z_1)**2.0)
return d_rad
class Ui_window1(object):
def setupUi(self, window1):
window1.setObjectName("window1")
window1.resize(485, 530) # 820 530
self.centralwidget = QtWidgets.QWidget(window1)
self.centralwidget.setObjectName("centralwidget")
window1.setCentralWidget(self.centralwidget)
self.groupBox_2 = QtWidgets.QGroupBox("groupBox_2", self.centralwidget)
self.output_rd = QtWidgets.QTextBrowser(self.groupBox_2)
self.output_rd.setGeometry(QtCore.QRect(10, 90, 331, 111))
self.output_rd.setObjectName("output_rd")
self.retranslateUi(window1)
QtCore.QMetaObject.connectSlotsByName(window1)
def retranslateUi(self, window1):
_translate = QtCore.QCoreApplication.translate
window1.setWindowTitle(_translate("window1", "GUI"))
def rad_distance(self):
time_rd = np.asarray([1, 2]) # ? (self.get_time_rd())
body1, body2 = ['EARTH', 'SUN']
rad_dis = radial_distance(body1, body2, time_rd, 'HCI', 'NONE', 'SUN')
# self.output_rd.setText(rad_dis)
self.output_rd.append(str(rad_dis)) # + str
if __name__ == "__main__":
import sys
app = QtWidgets.QApplication(sys.argv)
window1 = QtWidgets.QMainWindow()
ui = Ui_window1()
ui.setupUi(window1)
ui.rad_distance() # +
window1.show()
sys.exit(app.exec_())
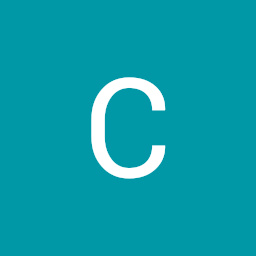
Author by
mrcfm4
Updated on June 04, 2022Comments
-
mrcfm4 almost 2 years
I'm coding a GUI which has a function that prints output to terminal. I would like to have it shown in some kind of text box or something similar.
Code looks like this:
def radial_distance(body1, body2, utc, ref, abcorr, obs): x_1 = 1 x_2 = 4 y_1 = 5 y_2 = 2 z_1 = 7 z_2 = 6 d_rad = np.sqrt((x_2 - x_1)**2.0 + (y_2 - y_1)**2.0 + (z_2 - z_1)**2.0) return d_rad
I've tried Text Label but I need more space to print (need to scroll down). Also tried Text Browser and Text Edit but didn't find the way to display it.
PyQt thing looks like:
from Distances import radial_distance from PyQt5 import QtCore, QtGui, QtWidgets class Ui_window1(object): def setupUi(self, window1): window1.setObjectName("window1") window1.resize(485, 530) # 820 530 self.centralwidget = QtWidgets.QWidget(window1) self.centralwidget.setObjectName("centralwidget") self.output_rd = QtWidgets.QTextBrowser(self.groupBox_2) self.output_rd.setGeometry(QtCore.QRect(10, 90, 331, 111)) self.output_rd.setObjectName("output_rd") def retranslateUi(self, window1): _translate = QtCore.QCoreApplication.translate window1.setWindowTitle(_translate("window1", "GUI")) def rad_distance(self): time_rd = np.asarray(self.get_time_rd()) body1, body2 = ['EARTH', 'SUN'] rad_dis = radial_distance(body1, body2, time_rd, 'HCI', 'NONE', 'SUN') self.output_rd.setText(rad_dis) # This doesnt work if __name__ == "__main__": import sys app = QtWidgets.QApplication(sys.argv) window1 = QtWidgets.QMainWindow() ui = Ui_window1() ui.setupUi(window1) window1.show() sys.exit(app.exec_())
Could you help me out? Thanks!
-
Ryan Schaefer over 4 yearsadd the code you have already
-
mrcfm4 over 4 years@RyanSchaefer there it is. I also tried to return d_rad and call it with self.label.setText(d_rad), but didn't work :(
-
mrcfm4 over 4 years@S.Nick i don't know if that's what you want because i got a lot of functions but i guess it sums everything up
-
-
mrcfm4 over 4 yearsThank you very much, that works. Is there any way to add more characters to the string? (for example units such as kilometers, etc)
-
S. Nick over 4 years@mrcfm4
self.output_rd.append("{} <-> {}".format(str(rad_dis), "kilometers"))
-
mrcfm4 over 4 yearsyou are the closest thing to god i've ever experienced, thank you very much (if you figure out a way to insert linejumps \n or whatever i promise i'll love you forever)
-
S. Nick over 4 years@mrcfm4
self.output_rd.append(""" {} <-> {} \n or whatever i promise i'll love you forever \n {})""".format( str(rad_dis), "kilometers", "Hello World!") )
-
mrcfm4 over 4 yearsyou are the smartest person in the universe, thank you very very much