How to return mouse coordinates in realtime?
13,176
The QMouseEvent function must be implemented since it is executed when the mouse is moved.
import sys
from PyQt5.QtWidgets import (QApplication, QLabel, QWidget)
class MouseTracker(QWidget):
def __init__(self):
super().__init__()
self.initUI()
self.setMouseTracking(True)
def initUI(self):
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('Mouse Tracker')
self.label = QLabel(self)
self.label.resize(200, 40)
self.show()
def mouseMoveEvent(self, event):
self.label.setText('Mouse coords: ( %d : %d )' % (event.x(), event.y()))
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MouseTracker()
sys.exit(app.exec_())
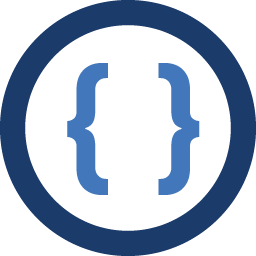
Author by
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I'm new to PyQt and I'm trying to use it to create a widget that returns the position of the mouse in real time.
Here's what I have:
import sys from PyQt5.QtWidgets import (QWidget, QToolTip, QPushButton, QApplication) from PyQt5.QtGui import QFont class MouseTracker(QWidget): def __init__(self): super().__init__() self.initUI() self.setMouseTracking(True) self.installEventFilter(self) def initUI(self): self.setGeometry(300, 300, 300, 200) self.setWindowTitle('Mouse Tracker') self.show() def eventFilter(self, source, event): if (event.type() == QtCore.QEvent.MouseMove and event.buttons() == QtCore.Qt.NoButton): pos = event.pos() print('Mouse coords: ( %d : %d )' % (pos.x(), pos.y())) return QtGui.QWidget.eventFilter(self, source, event) if __name__ == '__main__': app = QApplication(sys.argv) ex = MouseTracker() sys.exit(app.exec_())
I'm confused on how to make this work. I set mouse tracking to True but I'm not sure how to apply the event filter. Any help?