How can I get a char array in reverse order?
Solution 1
I believe what you wrote is the signature of the method you have to create.
public void printReverse(char[] letters, int size){
//code here
}
You would have to iterate the array and print what it contains backwards. Use a reverse "for loop" to go through each item in "letters". I'll let you combine these yourself as it's an assignment. Here's an example of a for loop:
for (int i = array.length-1; i >= 0 ; i--){
System.out.print(array[i]);
}
Solution 2
You can make use of StringBuilder#reverse() method like this:
String reverse = new StringBuilder(new String(letters)).reverse().toString();
Solution 3
This should take about 6 ms. It reverses a char array "in-place" before printing.
public static void reverseString(char[] s) {
int len = s.length;
if (len == 0)
return;
for (int i=0; i < (len/2); i++)
{
char l = s[i];
s[i] = s[len-i-1];
s[len-i-1] = l;
}
System.out.println(s);
}
Solution 4
`
//not only prints the reverse order, but creates new char array with chars in desired order
char[] letters = {'e', 'v', 'o', 'l', '4'};
int i = letters.length - 1, j = 0;
char[] let = new char[letters.length];
while(i >= 0){
let[j] = letters[i];
i--;
j++;
}
for (char c : let){
System.out.print(c);
}
`
output: 4love
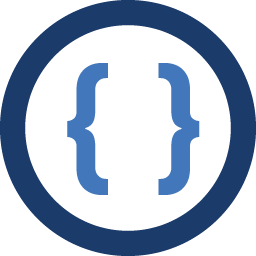
Admin
Updated on December 10, 2020Comments
-
Admin over 3 years
my assignment question is like that
Write a program which prints the letters in a char array in reverse order using
void printReverse(char letters[], int size);
For example, if the array contains {'c', 's', 'c', '2', '6', '1'} the output should be "162csc".
I tried, but I don't know what it means
void printReverse(char letters[], int size);
I did this but there's a problem with calling the method "printReverse" into the main method
import java.util.Arrays; import java.util.Collections; public class search { public static void main(String[] args) { char[] letters = {'e', 'v', 'o', 'l', '4'}; printReverse(); } public void printReverse(char[] letters, int size) { for (int i = letters.length-1; i >= 0 ; i--) { System.out.print(letters[i]); } }