How can I handle the change event with React-Hook-Form?
There is no onChange
method for Controller
as you defined in your code. So you can remove it this:
<Controller
control={control}
name="folderSelect"
onChange={() => console.log("hellow")} <- this one not required
defaultValue=""
What I understand for your question you want to trigger handleChange
as soon as select
value updated. For this you can do this:
<Select
labelId="demo-simple-select-label"
id="demo-simple-select"
onChange={(e) => {
onChange(e)
handleChange(e) <- call handleChange
}}
value={value ? value : ""}
name={name}
>
Here is code and demo: https://codesandbox.io/s/react-hook-form-select-onchange-forked-eqb20?file=/src/App.js:1533-1907
Related videos on Youtube
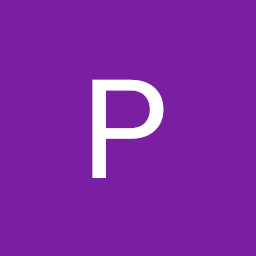
Paul McInerney
Updated on June 04, 2022Comments
-
Paul McInerney almost 2 years
I am trying to figure out onChange event using react hook form controller for materialui select but I cannot figure out how to trigger the event when select changes to call handleChange event. I have created a sandbox to replicate where I also have separate issue of
Warning: findDOMNode is deprecated in StrictMode
which I can't get my head around how to use the createRef to stop this but the main problem is onChange event as I need to render different further TextFields based off the Select-value.https://codesandbox.io/s/react-hook-form-select-onchange-uiic6
<form onSubmit={handleSubmit(onSubmit)}> <Grid container direction="column" spacing={2}> <Grid item> <FormControl fullWidth variant="outlined" className={classes.formControl}> <InputLabel id="demo-simple-select-label">Folder Name</InputLabel> <Controller control={control} name="folderSelect" onChange={handleChange} defaultValue="" render={({onChange, value, onBlur, name}) => ( <Select labelId="demo-simple-select-label" id="demo-simple-select" onChange={onChange} value={value ? value : ''} name={name}> <MenuItem value="Invoices" key="Invoices"> Invoices </MenuItem> <MenuItem value="Statements" key="Statements"> Statements </MenuItem> <MenuItem value="Credits" key="Credits"> Credits </MenuItem> </Select> )} /> </FormControl> </Grid> <Grid item> <TextField fullWidth label="First Name" name="firstName" variant="outlined" onChange={(e) => console.log(e.target.value)} inputRef={register({required: true})} /> </Grid> <Button type="submit">Submit</Button> </Grid> </form>;
-
Paul McInerney over 3 yearsthanks for input i know i can remove strictmode but i assumed its best not to that its there for a reason? tried using useRef createRef but to be honest it was just chancing it would work dont really understnad it,