Overriding the material-UI style not working
Solution 1
First of all, if you check the DOM structure
<div class="MuiTabs-root Tabs" aria-label="disabled tabs example">
<div class="MuiTabs-scroller MuiTabs-fixed" style="overflow: hidden;">
<div class="MuiTabs-flexContainer" role="tablist">
</div>
</div>
</div>;
You would find out that the demand className is MuiTabs-flexContainer (rather than tabFlexContainer
)
Example: For Tabs, all the className can be found in the MUI Tabs CSS API
There are many solutions, except normal withStyles
and makeStyles
, for fully override:
1.Material-UI solution
1.1 Use MUI internal style HOC withStyles to fully override the component.
Using nesting selector
import { Tabs, Tab, withStyles } from "@material-ui/core";
const StyledTabs = withStyles({
root: {
background: "light-blue",
...
boxShadow: "0 3px 5px 2px rgba(255, 105, 135, .3)",
"& div.MuiTabs-scroller": {
"& .MuiTabs-flexContainer": {
background: "linear-gradient(45deg, #FE6B8B 30%, #FF8E53 90%)"
}
}
}
})(Tabs);
1.2 Use normal createStyles or makeStyles style solution
Classical component
withStyles (High order function) + createStyles
Functional component
useStyles (hooks) + makeStyles
Refer in details: https://stackoverflow.com/a/60736142/11872246
2.Styled-Components solution
If you want to use separate CSS files to style MUI component
You can try styled-components
styled-components allows you to write actual CSS code to style your components.
Usage:
import styled from 'styled-components';
import { Tabs, Tab, withStyles } from "@material-ui/core";
const StyledTabs = styled.Tabs`
font-size: 1.5em;
...
`;
3.Separate Pure CSS solution
Refer: css_selectors
import "./styles.css";
div.MuiTabs-flexContainer {
background: linear-gradient(45deg, red 30%, #ff8e53 90%);
}
Solution 2
You can use the api(props) provided to tabs
by Material-UI here. like this:
import { makeStyles } from "@material-ui/styles";
const useStyles = makeStyles(theme => ({
tabRoot: {
// ...
},
flexContainer: {
width : 100%
justifyContent :"space-between"
}
}));
export default useStyles;
Use in your component:
const classes = useStyles();
// ...
<Tabs
classes={{ flexContainer: classes.flexContainer }} // override for tabs
...
>
<Tab classes={{ root: classes.tabRoot}} /> // override for tab
</Tabs>
See CSS section of these links.(Tab, Tabs)
Or you can use className
prop to add css class and override the styles.
Note: It's css in js, then style must be an object(camelCase property) not like css.
Solution 3
It is very hard to override Material UI using CSS but you can use either Styled-Components
or makeStyle
Hook.
Related videos on Youtube
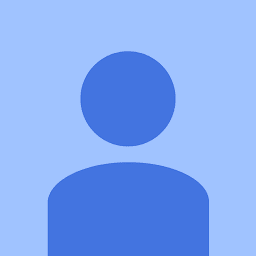
ganesh kaspate
Updated on August 15, 2022Comments
-
ganesh kaspate over 1 year
I am new to the material UI. Here, I am trying to override the CSS of material UI
tabs component.
<Tab key={`${tab}_${index}`} classes={{ flexcontainer: css.tabFlexContainer }} disableRipple label={tab.label} value={tab.value} icon={ tab.icon ? <Icons className={css.tabIcons} iconname={tab.icon} /> : null } />
So, here I am trying to override the
flexContainer
class with this CSS:. tabFlexContainer { width : 100% justify -content :space-between }
So, when I am using I am getting a compiled time error only,
TS2769: No overload matches this call.
Can anyone help me with this?
-
ganesh kaspate about 4 yearsI really appreciate your work , but will it possible if we do it the way I mean I have a seperate CSS file and there
-
ganesh kaspate about 4 yearsthing is this the way I am following from the start so , could u please help a bit . I got stuck over here. :-)
-
ganesh kaspate about 4 yearsSorry But I am not using the styled component for this
-
ganesh kaspate about 4 yearsActually, I am trying to use the classes props through which I can override the default css property.
-
keikai about 4 yearsYou forget a
s
formakeStyles
-
ganesh kaspate about 4 yearsawsome solution man. thank for your efforts . I do have one issue stackoverflow.com/questions/60931950/…. could u please look at it,
-
Hillsie almost 3 yearsFor me, this solution was easiest to implement. And if you want to override another prop
js ... classes={{ flexContainer: classes.flexContainer , indicator:classes.newIndicatorClass}} ...