ReactJS + Material-UI: How to alternate colors between Material-UI <Table/>'s <TableRow/>?
Solution 1
Try this. This is working fine in @version 4.4.2
{
this.state.data.map((row,index)=> (
<TableRow style ={ index % 2? { background : "#fdffe0" }:{ background : "white" }}>
...
</TableRow>
))}
Hope this will help you. Happy Coding...!
Solution 2
You can create a styled TableRow using withStyles and apply the even and odd css rules.
using Typescript:
const StyledTableRow = withStyles((theme: Theme) =>
createStyles({
root: {
'&:nth-of-type(odd)': {
backgroundColor: "white",
},
'&:nth-of-type(even)': {
backgroundColor: "grey",
},
},
}),
)(TableRow);
using Javascript:
const StyledTableRow = withStyles((theme) => ({
root: {
'&:nth-of-type(odd)': {
backgroundColor: "white",
},
'&:nth-of-type(even)': {
backgroundColor: "grey",
},
},
}))(TableRow);
And using your example, this is how it should be:
render(){
return(
<Table multiSelectable={true} >
<TableHeader>
<TableRow>
...
</TableRow>
</TableHeader>
<!-- stripedRows is no longer available in the newer versions of MUI -->
<TableBody displayRowCheckbox={true} >
<StyledTableRow>
...
</StyledTableRow>
...
This is how Material-UI does their odd/even row style in their docs.
You can see this working in this CodeSandbox example.
Solution 3
I'm a bit late, but for some reason stripedRows
didn't work for me, which is why i did it that way:
Just use the modulo operator to switch between 2 colors
{this.state.data.map((row)=> (
<TableRow style ={ row.rank % 2? { background : "#fdffe0" }:{ background : "white" }}>
...
</TableRow>
))}
@Version 4.2.1
Solution 4
You can use stripedRows
prop for <TableBody>
component to make the visual separation of rows, but I'm not sure that you can customise colors for this option.
<TableBody stripedRows > </TableBody>
Or you can achieve it by setting a className
for <TableBody>
component, and set colors with css even and odd rules. Probably, you should also set !important
for those rules to override inline styles.
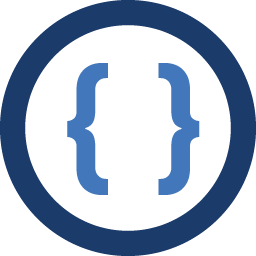
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I currently have a Material-UI's
<Table/>
( http://www.material-ui.com/#/components/table ), and would like each row to switch off between color blue and purple. So first one would be blue, then next row would be purple, and so on for any additional row added.How can I dynamically switch off between two colors for any additional rows added?
render(){ return( <Table multiSelectable={true} > <TableHeader> <TableRow> <TableHeaderColumn>First Name</TableHeaderColumn> <TableHeaderColumn>Last Name</TableHeaderColumn> <TableHeaderColumn>Color</TableHeaderColumn> </TableRow> </TableHeader> <TableBody displayRowCheckbox={true} stripedRows > <TableRow> <TableRowColumn>John</TableRowColumn> <TableRowColumn>Smith</TableRowColumn> <TableRowColumn>Red</TableRowColumn> </TableRow> <TableRow> <TableRowColumn>Paul</TableRowColumn> <TableRowColumn>Row</TableRowColumn> <TableRowColumn>Black</TableRowColumn> </TableRow> <TableRow> <TableRowColumn>Doe</TableRowColumn> <TableRowColumn>Boe</TableRowColumn> <TableRowColumn>Pink</TableRowColumn> </TableRow> <TableRow> <TableRowColumn>Frank</TableRowColumn> <TableRowColumn>Done</TableRowColumn> <TableRowColumn>White</TableRowColumn> </TableRow> <TableRow> <TableRowColumn>Lucy</TableRowColumn> <TableRowColumn>Ju</TableRowColumn> <TableRowColumn>Orange</TableRowColumn> </TableRow> </TableBody> </Table>
Thank you in advance
-
Admin over 7 yearsBefore I accept the answer and upvote, do you know if it's possible to change the color with
stripedRows
? Because that is the best approach and tried searching for it but no luck on the customization... -
Alexandr Lazarev over 7 yearsI didn't found a way to do this. You can try customise theme, but I don't think that's the case, since color will be changed everywhere. I've also opened an issue on github, to discuss this situation with maintainers. You cant track it down, because I might be wrong and there is a solution. github.com/callemall/material-ui/issues/5286
-
Admin over 7 yearsThank you so much! Will keep an eye out. One last question, upon selecting a checkbox, is there a way to get rid of the row highlight?
-
Alexandr Lazarev over 7 yearsNot sure I've understood you correctly. Do you mean to cancel striped property for all the rows or just for one?
-
Alexandr Lazarev over 7 yearsmaterial-ui.com/#/components/table here in second example you can toggle
stripedRows
prop for the table. Might be helpful example. -
Admin over 7 yearsNo worries. When I click the checkbox and check it off, the row gets highlighted. Was wondering how to get rid of the highlight even if I checked off the checkbox.
-
Alexandr Lazarev over 7 yearsWhat checkbox are you talking about?:) I don't see any checkboxes in the code sample you've provided.
-
Admin over 7 years
displayRowCheckbox
for the TableBody. Just forgot to include it :D -
Admin over 7 yearsplease let me know if you've seen my last comment.
-
Alexandr Lazarev over 7 yearsLet us continue this discussion in chat.
-
edwardrbaker over 4 yearsSometime between 2016 and 2019, this has changed and "stripedRows" is no longer available. Instead, you can do something similar:
{rows.map((row, index) => ( <TableRow key={row.percent} className={index%2 ? classes.lightRow : classes.darkRow}> ))}
-
yBrodsky over 2 yearsThis is the right answer. Give this man the ten thousand dollars