how can i ignore white space while scanning
14,934
From here:
"A Scanner breaks its input into tokens using a delimiter pattern, which by default matches whitespace".
So, your program splits the file according to whitespace first, and then .split(";");
splits these by ;
.
You need to set the delimiter to ;
as follows:
Scanner s = new Scanner(new File(testCase.getFileName())));
s.useDelimiter(";");
while (s.hasNext()) {
System.out.println(s.next());
}
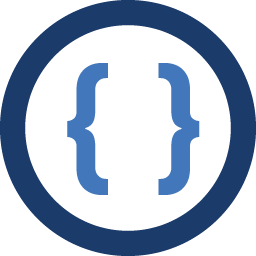
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I have a file:
12345678;ABC 123456A12345678;45678945
This is what I do:
Scanner s = new Scanner(new File(testCase.getFileName())); while (s.hasNext()) { String[] lineItems = s.next().split(";"); }
Output:
12345678;ABC 123456A12345678; 45678945
Desired output:
12345678 ABC 123456A12345678 45678945
I want it to consider
"ABC 123456A12345678"
as one single token and not break when it encounters whitespace.What should I do?