How can I make a copy of a file in Google Drive via Python?
Solution 1
There are a few tutorials around the web that give partial answers. Here is a step-by-step guide of what you need to do.
- Open Command prompt and type (without the quotes) "pip install PyDrive"
- Follow the instructions here by step one - https://developers.google.com/drive/v3/web/quickstart/python to set up an account
- When that is done, click on Download JSON and a file will be downloaded. Make sure to rename that to client_secrets.json, not client_secret.json as the Quick Start says to do.
- Next, make sure to put that file in the same directory as your python script. If you are running the script from a console, that directory might be your username directory.
- I assume that you already know the folder id that you are placing this file in and file id that you are copying. If you don't know it, there are tutorials of how to find it using python or you can open it up in Docs and it will be in the URL of the file. Basically enter the ID of the folder and the ID of the file and when you run this script it will make a copy of the chosen file and place it in the chosen folder.
- One thing to note is that while running, your browser window will open up and ask for permission, just click accept and then the script will complete.
- In order for this to work you might have to enable the Google Drive API, which is in the API's section.
Python Script:
## Create a new Document in Google Drive
from pydrive.auth import GoogleAuth
from pydrive.drive import GoogleDrive
gauth = GoogleAuth()
gauth.LocalWebserverAuth()
drive = GoogleDrive(gauth)
folder = "########"
title = "Copy of my other file"
file = "############"
drive.auth.service.files().copy(fileId=file,
body={"parents": [{"kind": "drive#fileLink",
"id": folder}], 'title': title}).execute()
Solution 2
From https://developers.google.com/drive/v2/reference/files/copy
from apiclient import errors
# ...
def copy_file(service, origin_file_id, copy_title):
"""Copy an existing file.
Args:
service: Drive API service instance.
origin_file_id: ID of the origin file to copy.
copy_title: Title of the copy.
Returns:
The copied file if successful, None otherwise.
"""
copied_file = {'title': copy_title}
try:
return service.files().copy(
fileId=origin_file_id, body=copied_file).execute()
except errors.HttpError, error:
print 'An error occurred: %s' % error
return None
Solution 3
With API v3:
Copy file to a directory with different name.
service.files().copy(fileId='PutFileIDHere', body={"parents": ['ParentFolderID'], 'name': 'NewFileName'} ).execute()
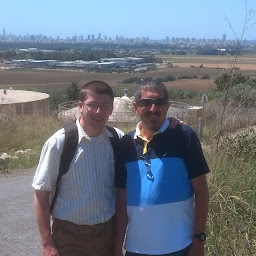
Zachary Weixelbaum
My favorite language is C++. Nowadays most people don't seem to care about speed because so many computers are fast enough, but if you want to get a program to be as fast as possible, but still using a real language (as opposed to machine language), C++ is the way to go. If you feel the need to use a higher level language with more functionality, C# is still better than Java! Don't use Java.
Updated on June 12, 2022Comments
-
Zachary Weixelbaum almost 2 years
I wrote a short function in Google Apps script that can make a copy of a specific file that is stored on Google Drive. The purpose of it is that this file is a template and every time I want to create a new document for work I make a copy of this template and just change the title of the document. The code that I wrote to make a copy of the file and store it in the specific folder that I want is very simple:
function copyFile() { var file = DriveApp.getFileById("############################################"); var folder = DriveApp.getFolderById("############################"); var filename = "Copy of Template"; file.makeCopy(filename, folder); }
This function takes a specific file, based on ID and a specific folder based on ID and puts the copy entitles "Copy of Template" into that folder.
I have been searching all over and I cannot seem to find this. Is there a way to do the exact same thing, but using Python instead? Or, at the very least is there a way to have Python somehow call that function to run this function? I need this to be done in Python because I am writing a script that does many functions at once whenever I start a new project for work, such as creating a new document from template in Google Drive as well as other things that are not related to Google Drive at all and they can therefore not be done in Google Apps Script.