How can I match on, but exclude a regex pattern?
45,706
Solution 1
You can either use lookbehind (not in Javascript):
(?<=cID=)[^&]*
Or you can use grouping and grab the first group:
cID=([^&]*)
Solution 2
Generally speaking, to accomplish something like this, you have at least 3 options:
- Use lookarounds, so you can match precisely what you want to capture
- No lookbehind in Javascript, unfortunately
- Use capturing group to capture specific strings
- Near universally supported in all flavors
- If all else fails, you can always just take a
substring
of the match- Works well if the length of the prefix/suffix to chop is a known constant
References
Examples
Given this test string:
i have 35 dogs, 16 cats and 10 elephants
These are the matches of some regex patterns:
-
\d+ cats
->16 cats
(see on rubular.com) -
\d+(?= cats)
->16
(see on rubular.com) -
(\d+) cats
->16 cats
(see on rubular.com)- Group 1 captures
16
- Group 1 captures
You can also do multiple captures, for example:
-
(\d+) (cats|dogs)
yields 2 match results (see on rubular.com)- Result 1:
35 dogs
- Group 1 captures
35
- Group 2 captures
dogs
- Group 1 captures
- Result 2:
16 cats
- Group 1 captures
16
- Group 2 captures
cats
- Group 1 captures
- Result 1:
Solution 3
With JavaScript, you'll want to use a capture group (put the part you want to capture inside ()
) in your regular expression
var url = 'http://example.com/createSend/step4_1.aspx?cID=876XYZ964D293CF&snap=true&jlkj=kjhkjh&';
var match = url.match(/cID=([^&]*)/);
// ["cID=876XYZ964D293CF", "876XYZ964D293CF"]
// match[0] is the whole pattern
// match[1] is the first capture group - ([^&]*)
// match will be 'false' if the match failed entirely
Solution 4
By using capturing groups:
cID=([^&]*)
and then get $1:
87B6XYZ964D293CF
Solution 5
Here's the Javascript code:
var str = "http://example.com/createSend/step4_1.aspx?cID=876XYZ964D293CF&snap=true&jlkj=kjhkjh&";
var myReg = new RegExp("cID=([^&]*)", "i");
var myMatch = myReg.exec(str);
alert(myMatch[1]);
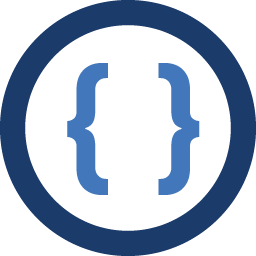
Author by
Admin
Updated on December 25, 2020Comments
-
Admin over 3 years
I have this URL:
http://example.com/createSend/step4_1.aspx?cID=876XYZ964D293CF&snap=true&jlkj=kjhkjh&
And this regex pattern:
cID=[^&]*
Which produces this result:
cID=87B6XYZ964D293CF
How do I REMOVE the "cID="?
Thanks
-
spex over 7 yearsThis is excessive.
'http://example.com/createSend/step4_1.aspx?cID=876XYZ964D293CF&snap=true&jlkj=kjhkjh&'.match(/cID=+([^&]*)/)[1];
is simpler as it does not require the non-capturing group and returns the same result as your example.