How can I reload a jQuery dialog box from within the dialog?
Solution 1
You are loading content from another page into the dialog container in the current page, so there is really only one page in play here. I would recommend only loading partial html (no <head>
or <body>
tags) from your dialog source page.
So you can either destroy the dialog and recreate it to reload the dialog content:
function reload_dialog()
{
$('#dialog').dialog('destroy');
view_dialog();
}
function view_dialog() {
$('#dialog').load('blah2.aspx').dialog({
title: 'test' ,
modal: 'true',
width: '80%',
height: '740',
position: 'center',
show: 'scale',
hide: 'scale',
cache: false });
}
Or, you can reload the entire page.
window.location.reload();
Solution 2
You can extend the ui.dialog in jQuery like this:
$.ui.dialog.prototype.options.url; // Define a new option 'url'
Now, you can replace the entire dialog "create" so it loads the url automatically and define the new "refresh" option:
// Replace the _create event
var orig_create = $.ui.dialog.prototype._create;
$.ui.dialog.prototype._create = function(){
orig_create.apply(this,arguments);
var self = this;
if(this.options.url!=''){
self.element.load(self.options.url); // auto load the url selected
};
};
$.widget( "ui.dialog", $.ui.dialog, {
refresh: function() {
if(this.options.url!=''){
this.element.load(this.options.url);
}
}
});
Your final function would look like this:
// Note the "url" option.
function view_dialog() {
$('#dialog').dialog({
url:'blah2.aspx',
title: 'test' ,
modal: 'true',
width: '80%',
height: '740',
position: 'center',
show: 'scale',
hide: 'scale',
cache: false });
}
// refresh:
$('#dialog').dialog("refresh");
You can see the final code in this fiddle: http://jsfiddle.net/WLg53/1/
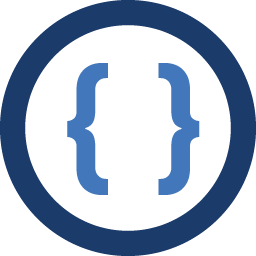
Admin
Updated on June 04, 2020Comments
-
Admin almost 4 years
I load a page within a jQuery
Dialog
. After an ajax command is completed, I would like to be able to reload the same page within the jQueryDialog
. How can I reload a jQueryDialog
from within the page that is loaded in theDialog
?Parent Page (Blah.aspx):
<script type="text/javascript"> function view_dialog() { $('#dialog').load('blah2.aspx').dialog({ title: 'test' , modal: 'true', width: '80%', height: '740', position: 'center', show: 'scale', hide: 'scale', cache: false }); } </script> <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server"> <input id="Button1" onclick="view_dialog()" type="button" value="button" /> <div id="dialog" style="display: none"></div> </asp:Content>
Dialog contents page (blah2.aspx):
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server"> <script type="text/javascript"> $(document).ready(function() { function doit() { var request = $.ajax({ url: "someurl", dataType: "JSON", data: { a: 'somevalue' } }); request.done(function () { //refresh the current dialog by calling blah2.aspx again }); } </script> <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="server"> <input id="Button1" onclick="doit()" type="button" value="button" /> </asp:Content>
-
Markoh over 7 yearsThank you for this! I know it's over 4 years later, but your code helped me out immensely!