How can I save data from a Windows Form to an XML file?
Solution 1
One simple way to do this would be to create .NET classes that you put the data in and then use XmlSerializer to serialize the data to a file and then later deserialize back into an instance of the class and re-populate the form.
AS an example, if you have a form with customer data. To keep it short we will just have first name and last name. You can create a class to hold the data. Keep in mind this is just a simple example, you can store arrays and all kinds of complex/nested data like this.
public class CustomerData
{
public string FirstName;
public string LastName;
}
So save the data as XML your code will look something like this.
// Create an instance of the CustomerData class and populate
// it with the data from the form.
CustomerData customer = new CustomerData();
customer.FirstName = txtFirstName.Text;
customer.LastName = txtLastName.Text;
// Create and XmlSerializer to serialize the data to a file
XmlSerializer xs = new XmlSerializer(typeof(CustomerData));
using (FileStream fs = new FileStream("Data.xml", FileMode.Create))
{
xs.Serialize(fs, customer);
}
And loading the data back would be something like the following
CustomerData customer;
XmlSerializer xs = new XmlSerializer(typeof(CustomerData));
using (FileStream fs = new FileStream("Data.xml", FileMode.Open))
{
// This will read the XML from the file and create the new instance
// of CustomerData
customer = xs.Deserialize(fs) as CustomerData;
}
// If the customer data was successfully deserialized we can transfer
// the data from the instance to the form.
if (customer != null)
{
txtFirstName.Text = customer.FirstName;
txtLastName.Text = customer.LastName;
}
Solution 2
look at using Linq to xml - http://msdn.microsoft.com/en-us/library/bb387098.aspx there are tutorials here that will guide you through creating and querying an xml document.
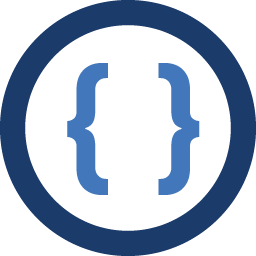
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I pretty sure I have to create some sort of mold of what the XML file has to look like first, right?
Any help will be appreciated.
-
Aren almost 14 years+1 This is the method I would suggest. The only problem you may encounter is that if your data changes, old versions will not always deserialize to the DataObject correctly. You have to write a converter to update the XML to the new schema, or write an alternate loader for the xml (based on the old schema) and then re-save it with the
XmlSerializer
-
dlras2 almost 14 yearsIs there any reason you decided to use a
class
and not astruct
? -
Chris Taylor almost 14 years@cyclotis04, a struct is good for smallish immutable data. You end-up passing instances of the data classes around to other parts of the application so passing references is nice and efficient for large data structures. This is probably your typical struct vs. class discussion, and for me I normally go with classes unless I really understand the need and see the value of going with a struct, but that is just a rule of thumb for me.
-
Robert Fleck almost 11 yearsI would also like to piggy-back on this question and ask how you might work this solution with an array or list of data.
-
Chris Taylor almost 11 years@RobertFleck - Create a class which contains the array or list and then serialize that class. This leaves you with the possibility to add other data other than the array/list if ever required.