How can I style layer.control in leaflet.js?
Solution 1
Add this style after leaflet.css:
<style>
.leaflet-control-layers-toggle:after{
content:"your text";
color:#000 ;
}
.leaflet-control-layers-toggle{
width:auto;
background-position:3px 50% ;
padding:3px;
padding-left:36px;
text-decoration:none;
line-height:36px;
}
</style>
Solution 2
You can use HTML and you can change text when you define baselayers dans overlays.
Example:
var baseLayers = {
"<div id='my-div-id'><img src='img/icon-minimal.png' />Minimal View</div>": minimal,
"<div id='my-div-id'><img src='img/icon-night.png' />Super Night View</div>": midnight
};
var overlays = {
"<img src='myimage.png' /> Motorways": motorways,
"<img src='myimage2.png' /> All Cities": cities
};
Solution 3
If you are using Jquery you can add this to the document ready section
$(document).ready(function () {
$('.leaflet-control-layers').css({ 'width': 'auto', 'float': 'left' });
$('.leaflet-control-layers-toggle').css('float', 'left');
$('.leaflet-control-layers-toggle').after('<div class='control-extend'>Your text goes here</div>')
$('.control-extend').css({ 'float': 'left', 'line-height': '35px', 'font-weight': 'bold', 'margin-right' : '10px'});
$('.leaflet-control-layers').on('mouseover', function (e) {
$('.control-extend').hide();
});
$('.leaflet-control-layers').on('mouseout', function (e) {
$('.control-extend').show();
});
});
Solution 4
This actually doesn't work because there is apparently no way to control the vertical spacing between the radio buttons, which means that the buttons don't line up with the labels (and images).
The only example of this sort of thing I have seen that ACTUALLY WORKS is in the Leaflet Providers plug-in demo page in the package at https://github.com/leaflet-extras/leaflet-providers. What he apparently has done is to place the radio button and label on a overlay above the actual layer control, sort of like the Leaflet attribution overlay at the bottom right of most Leaflet maps...
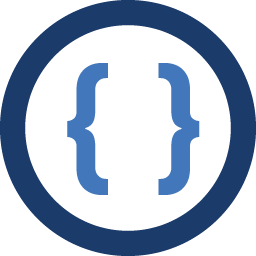
Admin
Updated on June 06, 2020Comments
-
Admin almost 4 years
I am trying to change the default dropdown menu icon in the layer control. I'd like to have text alongside the icon. Is there any way to do this? Perhaps using JQuery and CSS?
I'm working on a leaflet project based on this example: http://leafletjs.com/examples/layers-control.html
Code:
<html> <head> <title>Leaflet Layers Control Example</title> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="../dist/leaflet.css" /> </head> <body> <div id="map" style="width: 600px; height: 400px"></div> <script src="../dist/leaflet.js"></script> <script> var cities = new L.LayerGroup(); L.marker([39.61, -105.02]).bindPopup('This is Littleton, CO.').addTo(cities), L.marker([39.74, -104.99]).bindPopup('This is Denver, CO.').addTo(cities), L.marker([39.73, -104.8]).bindPopup('This is Aurora, CO.').addTo(cities), L.marker([39.77, -105.23]).bindPopup('This is Golden, CO.').addTo(cities); var cmAttr = 'Map data © 2011 OpenStreetMap contributors, Imagery © 2011 Clou dMade', cmUrl = 'http://{s}.tile.cloudmade.com/BC9A493B41014CAABB98F0471D759707/{styleId}/256/{z}/{x}/{y}.png'; var minimal = L.tileLayer(cmUrl, {styleId: 22677, attribution: cmAttr}), midnight = L.tileLayer(cmUrl, {styleId: 999, attribution: cmAttr}), motorways = L.tileLayer(cmUrl, {styleId: 46561, attribution: cmAttr}); var map = L.map('map', { center: [39.73, -104.99], zoom: 10, layers: [minimal, motorways, cities] }); var baseLayers = { "Minimal": minimal, "Night View": midnight }; var overlays = { "Motorways": motorways, "Cities": cities }; L.control.layers(baseLayers, overlays).addTo(map); </script>
-
Jeff over 9 yearsThanks. Too bad OP didn't bother choosing this as the answer. How would I go about changing that "after" content via jQuery?
-
Dr.Molle over 9 years@Jeff:
jQuery(function($){$('.leaflet-control-layers-toggle').append('some text');});