How can I use JavaScript to limit a number between a min/max value?
Solution 1
like this
var number = Math.min(Math.max(parseInt(number), 1), 20);
Live Demo:
function limitNumberWithinRange(num, min, max){
const MIN = min || 1;
const MAX = max || 20;
const parsed = parseInt(num)
return Math.min(Math.max(parsed, MIN), MAX)
}
alert(
limitNumberWithinRange( prompt("enter a number") )
)
Solution 2
You have at least two options:
You can use a pair of conditional operators (? :
):
number = number > 100 ? 100 : number < 0 ? 0 : number;
Or you can combine Math.max
and Math.min
:
number = Math.min(100, Math.max(0, number));
In both cases, it's relatively easy to confuse yourself, so you might consider having a utility function if you do this in multiple places:
function clamp(val, min, max) {
return val > max ? max : val < min ? min : val;
}
Then:
number = clamp(number, 0, 100);
Solution 3
Use lodash's clamp
method:
_.clamp(22, 1, 20) // Outputs 20
Solution 4
Needs no further explanation:
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
}
Solution 5
Related: What's the most elegant way to cap a number to a segment?:
Update for ECMAScript 2017:
Math.clamp(x, lower, upper)
But note that as of today, it's a Stage 1 proposal. Until it gets widely supported, you can use a polyfill.
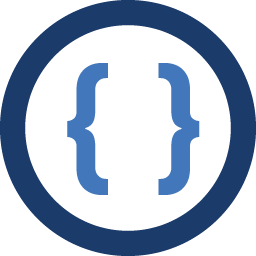
Admin
Updated on December 24, 2021Comments
-
Admin over 2 years
I want to limit a number between two values, I know that in PHP you can do this:
$number = min(max(intval($number), 1), 20); // this will make $number 1 if it's lower than 1, and 20 if it's higher than 20
How can I do this in javascript, without having to write multiple
if
statements and stuff like that? Thanks. -
Harry Joy about 13 yearsdo Javascript has as
intval(num)
function. Cause I know onlyparseInt(num)
? -
Govind Malviya about 13 yearsI dont know about php sorry I edited that I consider it as User defined function
-
Harry Joy about 13 yearsNow our answers are identical so I'm deleting mine.
-
Govind Malviya about 13 yearsthanks Joy for telling me about intval in php. and thanks for providing chance to me..
-
Oriol over 8 yearsDon't use
parseInt
unless you know what you are doing, e.g.parseInt(1e300) === 1
. Just useMath.min(Math.max(number, 1), 20)
. -
Sandwich almost 8 yearsIsn't this backwards? As written, the number is maximum 1, and minimum 20, no?
-
Константин Ван almost 8 years@Sandwich Let's pay attention to
Math.max(number, 1)
. It's the maximum value amongnumber
and1
. So it'snumber
whennumber > 1
, and it's1
whennumber <= 1
. i.e. it is always greater than or equals to1
, no matter whatnumber
is, as long asnumber
is numeric(Math.max
can returnNaN
whennumber
is not numeric.); theMath.max
sets the minimum limit to1
. And theMath.min
sets the maximum limit to20
in the same way. -
Константин Ван almost 8 years@Sandwich Remember,
Math.min
andMath.max
are notMath.setMinimumLimit
andMath.setMaximumLimit
. Don't be confused. -
Leonardo Raele over 5 yearsThat's what I was looking for. Thank you
-
Ryan Stone over 3 yearsWould this work for negative numbers aswell such as transform -xdeg?
-
Neuron over 3 years@RyanStone what do you mean by "transform -xdeg"? It does work for negative values as well, yes. You can call
clamp(-5, -1, 1)
and it will return-1
. -
Ryan Stone over 3 yearswas meaning to use it in Css Transform:rotateX(); which takes in negative values.. Via Javascript