How can I use provider without context?
I’m assuming that your _buildName()
is a method of your widget and that you call it from you build method somewhere.
This means that you can pass either the context or the user into this method:
_buildName(BuildContext context) {}
or _buildName(User user) {}
Try providing more of the code next time, specifically the parts where you call the method.
Edit after post update:
You need to have the user object in the buildName method so you cannot simply do UserData.fullname
because UserData
is a class not an instance.
So to get your data in the buildName you need to change it to:
_buildName(UserData userData) {
userData.fullname; // this now exists
}
And call is like: _buildName(userData)
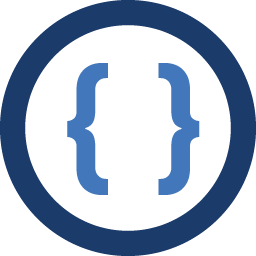
Admin
Updated on December 12, 2022Comments
-
Admin over 1 year
I have a widget where I use the provider but where I want to use the values is in normal widgets so maybe anyone can help.
This is where I use the provider :
Widget _buildName(BuildContext context) { return Column( crossAxisAlignment: CrossAxisAlignment.start, children: <Widget>[ Text( 'Full Name', style: kLabelStyle3, ), SizedBox(height: 10.0), Container( alignment: Alignment.centerLeft, height: 50.0, child: TextFormField( initialValue: UserData.fullname; validator: (val) => val.isEmpty ? 'Enter your Name' : null, onChanged: (val) { setState(() => _currentfullname = val); }, style: TextStyle( color: Colors.black, fontFamily: 'OpenSans', ), decoration: InputDecoration( enabledBorder: OutlineInputBorder( borderRadius: BorderRadius.circular(25.0), borderSide: BorderSide( color: Colors.black, width: 2.0, ), ), contentPadding: EdgeInsets.only(top: 14.0), prefixIcon: Icon( Icons.person, color: Colors.black, ), hintText: 'Enter your Name', hintStyle: kHintTextStyle2, ), ), ), ], ); }
@override Widget build(BuildContext context) { final user = Provider.of<Userr>(context); return StreamBuilder<UserData>( stream: DatbaseService(uid:user.uid).userData, builder: (context, snapshot) { if(snapshot.hasData){ UserData userData =snapshot.data; return Scaffold( appBar: AppBar( backgroundColor: Colors.transparent, elevation: 0.0, ), body: AnnotatedRegion<SystemUiOverlayStyle>( value: SystemUiOverlayStyle.light, child: GestureDetector( onTap: () => FocusScope.of(context).unfocus(), child: Form( key: _formKey, child: Stack( children: <Widget>[ Container( height: double.infinity, child: SingleChildScrollView( physics: AlwaysScrollableScrollPhysics(), padding: EdgeInsets.symmetric( horizontal: 40.0, vertical: 10, ), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Center( child: Stack( children: [ Container( width: 110, height: 110, decoration: BoxDecoration( borderRadius: BorderRadius.circular(100), image: DecorationImage( fit: BoxFit.cover, image: NetworkImage( "https://images.pexels.com/photos/3307758/pexels-photo-3307758.jpeg?auto=compress&cs=tinysrgb&dpr=3&h=250", ))), ), Positioned( bottom: 0, right: 0, child: Container( height: 35, width: 35, decoration: BoxDecoration( shape: BoxShape.circle, border: Border.all( width: 4, color: Theme.of(context) .scaffoldBackgroundColor, ), color: Colors.green, ), child: Icon( Icons.edit, color: Colors.white, ), )), ], ), ), SizedBox( height: 10, ), Text( 'Mein Profil', style: TextStyle( color: Colors.black, fontFamily: 'OpenSans', fontSize: 20.0, fontWeight: FontWeight.w600, ), ), showAlert2(), _buildEmailTF(), SizedBox( height: 30.0, ), _buildName(), SizedBox( height: 30.0, ), _builduserName(), SizedBox( height: 30.0, ), _buildPasswordTF(), SizedBox(height: 30,), _buildPassword2TF(), _buildUpdateDataButton(), // _buildEmailform(), ], ), ), ) ], ), ), ), ), ); }else{ return null; } } ); } }
class DatbaseService{ final String uid; DatbaseService({this.uid}); //collection reference final CollectionReference myprofilsettings = FirebaseFirestore.instance.collection('meinprofilsettings'); Future updateUserData(String user,String fullname,String password,String email)async{ return await myprofilsettings.doc(uid).set({ 'username':user, 'fullname':fullname, 'passwort':password, 'email':email, }); } //profil list from snapshot List<myprofil> _myprofillistFromSnapshot(QuerySnapshot snapshot){ return snapshot.docs.map((doc){ return myprofil( user: doc.data()['user']??'', fullname: doc.data()['fullname']??'', email: doc.data()['email']??'', passowrd: doc.data()['password']??'', ); }).toList(); } //userData from snapshot UserData _userDataFromSnapshot(DocumentSnapshot snapshot){ return UserData( uid: uid, name: snapshot.data()['name'], fullname: snapshot.data()['fullname'], email: snapshot.data()['email'], password: snapshot.data()['password'], ); } //get myprofilsettings stream Stream<List<myprofil>> get settings{ return myprofilsettings.snapshots().map(_myprofillistFromSnapshot); } //get user doc stream Stream<UserData> get userData{ return myprofilsettings.doc(uid).snapshots().map(_userDataFromSnapshot); } }
import 'package:flutter/cupertino.dart'; class Userr{ final String uid; Userr({this.uid}); } class UserData { final String uid; final String user; final String fullname; final String email; final String passowrd; UserData({this.uid,this.user,this.fullname,this.email,this.passowrd, name, password});
Ignore this: Because flutter says __it looks like It looks like your post is mostly code; please add some more details. ___ IM adding some textdehpkfnwrfemrjfikerfoiwnfdoiwjefiojnweoidfjwiodjwiojdoijweiodjweiojdoiewjdijewoijdoejwdiojewiojdiowjedijweoidjiowediwjdoiwejdiowjdiojwoidjaldknjlncjnnc xy,,y,y,,y,ykampkdnndendiowendiojweiopjdipqejkdpojkdposkqwpodkqopwkdopkqwopdskqopdkpoqwkdopqkwopdkqwpodkpoqkdpkqpodkpqkdpokdpo<skcpoaskdpoakdopkdpoekwopdkwepokdpowekdpokwepodkwepokdpowekdpowekpdkpekdpokeopdkpekdpowekdopewkpdkwpekdpwekdpowekdpowekdpowekdpkwepodkwepodkpoekdpoewkdpoekdp
======== Exception caught by widgets library ======================================================= The following assertion was thrown building StreamBuilder<UserData>(dirty, state: _StreamBuilderBaseState<UserData, AsyncSnapshot<UserData>>#c612d): A build function returned null. The offending widget is: StreamBuilder<UserData> Build functions must never return null. To return an empty space that causes the building widget to fill available room, return "Container()". To return an empty space that takes as little room as possible, return "Container(width: 0.0, height: 0.0)". The relevant error-causing widget was: StreamBuilder<UserData> file:///Users/name/StudioProjects/project/lib/seitenleiste/meinacount.dart:356:16 When the exception was thrown, this was the stack: #0 debugWidgetBuilderValue.<anonymous closure> (package:flutter/src/widgets/debug.dart:305:7) #1 debugWidgetBuilderValue (package:flutter/src/widgets/debug.dart:326:4) #2 ComponentElement.performRebuild (package:flutter/src/widgets/framework.dart:4592:7) #3 StatefulElement.performRebuild (package:flutter/src/widgets/framework.dart:4759:11) #4 Element.rebuild (package:flutter/src/widgets/framework.dart:4281:5) ... ====================================================================================================
-
Steve about 3 yearsYou can't use Provider without a BuildContext. Consider using riverpod.dev instead. I hope this answer helps you, since the question is actually not clear enough.
-
Admin about 3 yearshmm not really can you maybe show on my code how to doing that ....
-
-
Admin about 3 yearsit make sense and look at my code you're right I call it in the widget . But how can I use this what im showing. I mean the initialvalue in my method _builName. when im using userData.fullname it shows undefined name
-
Pieter van Loon about 3 yearsPlease just include the whole widget, it’s not readable like this
-
Admin about 3 yearsThank you so much:)
-
Admin about 3 yearshey sorry agin but I have a error and think it not useful to create a new question I updating my maybe you can check. thanks !
-
Pieter van Loon about 3 yearsThe error explains what you did wrong, if you cannot figure it out open a new question
-
Admin about 3 yearsstackoverflow.com/questions/66591206/…. Please check