How do I access a method of the state objects for a list of stateful widgets? (Flutter)
689
You can use a GlobalKey with the Widget's state to access the child Widget's methods:
class Main extends StatefulWidget {
@override
_MainState createState() => _MainState();
}
class _MainState extends State<Main> {
GlobalKey<_HomeState> _key = GlobalKey();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('StackOverflow'),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Home(key: _key),
RaisedButton(
onPressed: () => _key.currentState.changeText('new text'),
child: Text('Change text'),
)
],
)
);
}
}
class Home extends StatefulWidget {
Home({Key key}) : super(key: key);
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
String text = 'initial text';
@override
Widget build(BuildContext context) {
return Center(
child: Text(text)
);
}
void changeText(String newText){
setState(() {
text = newText;
});
}
}
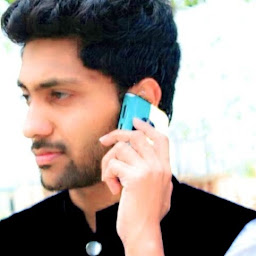
Author by
Nand gopal
Updated on December 16, 2022Comments
-
Nand gopal over 1 year
class Astate extends State<A> { List b=new List<B>(); @override Widget build(BuildContext context){ b.add(B()); //how do I access theMethod() for the state object for b[0]? } } class B extends StatefulWidget { @override Bstate createState() => Bstate(); } class Bstate extends State<B> { theMethod() { //some content } @override Widget build(BuildContext context) { } }
Is there a way to access the theMethod() using b[0] from it's corresponding state object? If not, is there another way to achieve the same?