How do i access ejs data in a form while using express
Let's start with getting the info into the form. Edit the form like so:
<form action="/clubreq" method="post">
<% for (var i in clubreq){%>
Club Name: <input type="text" name="clubname[]" value="<%= clubreq[i].clubname %>" /><br><br>
Club Type: <input type="text" name="clubtype[]" value="<%= clubreq[i].type %>" /><br><br>
<input type="submit" value="accept"/><br><br><hr>
<%} %>
</form>
The important points being adding the action attribute indicating where to submit the form and the addition of inputs to ensure the values and transported with the form. If you don't like the way it looks, you can change the input type to hidden and then add another echo like you had before of club name and club type for the end user to see.
Now on to how to access your form data server side, add the following to the top with all your other middleware:
app.use(express.bodyParser());
Next, you can access the post data in your method like so:
app.post('/clubreq', function(req, res, next){
// req.body object has your form values
console.log(req.body.clubname);
console.log(req.body.clubtype);
});
Hope that helps
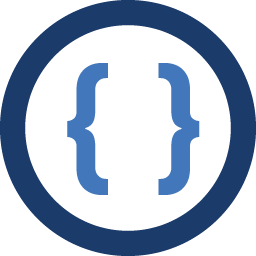
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
In the following code, I display the following HTML file upon user requests. I also want to access the clubname and type datavalues inside my app.post() function in my app.js file.
<!DOCTYPE html> <html> <head> <title><%= title %></title> <link rel='stylesheet' href='/stylesheets/style.css' /> </head> <body> <% include templates/adminheader.ejs %> <h1>Respond to Club Requests</h1> <form name="clubform" method="post"> <% for (var i in clubreq){%> Club Name:<%= clubreq[i].clubname %><br><br>//I want to access this variable in my app.post() function Club Type:<%= clubreq[i].type %><br><br> <input type="submit" value="accept"/><br><br><hr> <%} %> </form> </body> </html>
Inside app.js. Here I am rendering the HTML file upon user request
app.get('/clubreq', function(req, res, next){ res.render('clubreq', { title: 'Club Requests', "clubreq" : docs }); }); app.post('/clubreq', function(req, res, next){ //I want to access clubname and type datavariables here and store it in my mongodb database });