Node Ejs module, include not working
Solution 1
Express 3 breaks ejs partials, use express-partials
instead.
// Include it like so with your other modules
var express = require("express");
var partials = require('express-partials');
var server = express();
// Finally add it into your server conviguration
server.configure(function(){
server.set('view engine', 'ejs');
// Include partials middleware into the server
server.use(partials());
});
In your .ejs
views, enjoy the luxury like so...
<%- include ../partials/header %>
<section id="welcome">
Welcome
</section>
<%- include ../partials/footer %>
Also, rather than setting the ejs module to read .html
just follow this answer to get eclipse to render .ejs
as .html
. how do i get eclipse to interpret .ejs files as .html?
As an example, this is how a basic express structure is setup...
project
--public
--views
----layout.ejs
----index.ejs
----partials
------partial1.ejs
--server.js
server.js would look like...
var express = require('express'),
partials = require('express-partials'),
http = require('http'),
path = require('path');
var app = express();
// all environments
app.configure(function() {
app.set('port', process.env.PORT || 3838);
app.set('views', __dirname + '/views');
app.set('view engine', 'ejs');
// Middleware
app.use(partials());
app.use(express.favicon());
app.use(express.logger('dev'));
app.use(express.json());
app.use(express.urlencoded());
app.use(express.methodOverride());
app.use(app.router);
app.use(express.static(path.join(__dirname, 'public')));
});
http.createServer(app).listen(app.get('port'), function(){
console.log('App listening on port ' + app.get('port'));
});
Your route would look like...
server.get("/", function(req, res) {
res.render("index");
});
And finally, your layout.ejs...
<html>
<head>
<title></title>
</head>
<body>
<%- body %>
</body>
</html>
and index.ejs ...
<div id="index">
<%- include partial1.ejs %>
</div>
If you need a reference to the working example, here it is
Solution 2
With include you shoud do like this:
<%- include ("./partials/footer") %>
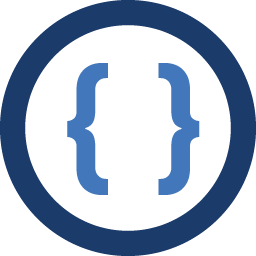
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Im having some trouble including a html snippets into my index.html . I have tried to follow, the ejs documentation but I just can't seem to make it work.
Directory Structure:
project -public --assets ---css ---images ---js --Index ---index.html + index.css and index.js --someOtherPageFolder -views --partials ---partials1.ejs --index.ejs --layout.ejs -server.js
This is my server.js (NEW):
var express = require("express"); var partials = require("express-partials"); var http = require('http'); var path = require('path'); var app = express(); app.get("/", function(req, res) { res.redirect("index"); }); app.configure(function(){ app.set('port', process.env.PORT || 8888); app.set('views', __dirname + '/views'); app.set('view engine', 'ejs'); app.use(partials()); app.use(express.favicon()); app.use(express.logger('dev')); app.use(express.json()); app.use(express.urlencoded()); app.use(express.methodOverride()); app.use(express.bodyParser()); app.use(app.router); app.use(express.static(path.join(__dirname, 'public'))); }); http.createServer(app).listen(app.get('port'), function(){ console.log('App listening on port ' + app.get('port')); });
This is my test.ejs:
<h1>This is test!</h1>
And this is where I want the html snipp to go:
<div id="sb-site"> <div class=""> <p>Not test</p> <%- include test.html %> </div> </div>
What am I doing wrong? Is there also a way for me to do this and use .html instead of .ejs (Im using eclipse and it doesn't support .ejs files)
-
Admin about 10 yearsthank you! But I still cant seem to make it work ? I have done all of the above but when I then go to the site it just downloads the index.ejs file. And I dont think I understand "in your .ejs views..". Do I need a seperate folder for my .ejs files? This is looking much better though :)
-
Seth about 10 yearsyes, did you add
server.set('views', __dirname + '/views');
in your config? You need to add a directory for your views to serve from. -
Admin about 10 yearsI have now added server.set('views', __dirname + '/views'); + server.use(express.static('public', __dirname + '/public')); My index.html is in my /public folder. Do I then include .ejs files from the /views folder?
-
Seth about 10 yearsYes! layouts and all views go in the views folder, refer to my edit, under "Your router would look like..." to see how to render the view.
-
Seth about 10 yearsBy all views, I mean all .ejs files reside in your views directory
-
Admin about 10 yearsokay thanks. I have done everyhting you have told me so far, but Im still not able to make it work. I think it may be because I dont understand what I need layout.ejs and partials1.ejs for or what my index is. Is my index and all my future web pages located in the "/views" folder? I can write down how my directory is set up in the original post and update all the code if you want to? btw, it still download the index.ejs file to my downloads folder instead of showing it in the browser when I got to localhost. Thank you for all your help so far! :D
-
Seth about 10 yearsNot a problem, thank YOU for choosing node.js. The directory structure would help immensely and any updates you've made to your server setup, please add to your original post.
-
Seth about 10 years