How do I add cookies in a post request in Java?
29,251
Solution 1
Here's a simple example of setting a cookie in a POST request with URLConnection
:
URL url = new URL("http://example.com/");
String postData = "foo bar baz";
URLConnection con = url.openConnection();
con.setDoOutput(true);
con.setRequestProperty("Cookie", "name=value");
con.setRequestProperty("Content-Type", "text/plain; charset=utf-8");
con.connect();
OutputStreamWriter out = new OutputStreamWriter(con.getOutputStream(), "UTF-8");
out.write(postData);
out.close();
You probably need to pass a cookie from a previous request, see this answer for an example. Also consider using Apache HttpClient to make things easier.
Solution 2
URL url = new URL("http://hostname:80");
URLConnection conn = url.openConnection();
conn.setRequestProperty("Cookie", "name1=value1; name2=value2");
conn.connect();
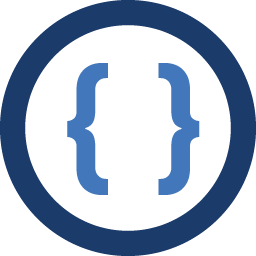
Author by
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
I was trying to get a certain page through java, but with this page I didn't succeed. Now in my browser it does work, but when I disable Cookies in the settings, it doesn't anymore.
So I probably need to add cookies to my post request in java.So I went searching the interwebs, but unfortunately I couldn't really find anything useful. mostly it was vague, scattered or irrelevant.
So now my question :
Could anyone show me how to do it (mentioned above^^), or point me to a clear site? -
Johan almost 5 yearsWhile this code snippet may be the solution, including an explanation really helps to improve the quality of your post. Remember that you are answering the question for readers in the future, and those people might not know the reasons for your code suggestion.