Spring Boot Content type 'text/plain' not supported in POST request in terminal
Please modify a couple of things:
- In case you expect a
Map
in the request body, you need to haveconsumes
content type something other thantext/plain
likeapplication/json
. Thetext/plain
content won't be converted toMap
by any converter. Otherwise, take request body asString
and internally convert it toMap
in your code. - In curl request add
-X POST
. Also, make the payload structure JSON key value pairs.
You are getting 405 error code due to text content in payload and expected request as Map
data type. To confirm this, just remove the request body Map
and see whether your API is hit. And then follow the steps above.
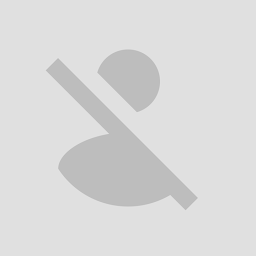
schooner_101
Interested in bioinformatics, AI, machine learning, and software development practices. Going to college to study computer science and seeking summer internships/research in software engineering and AI Development. Currently working at an private AI healthcare startup.
Updated on June 04, 2022Comments
-
schooner_101 almost 2 years
I have an application in spring boot. I created a post request to accept a string and to spit out a JSON in a text file. I am using @RequestBody as a Map. I'm not sure if I am utilizing that properly and that is the reason I am getting the error?
When I try to do
curl -d "ncs|56-2629193|1972-03-28|20190218|77067|6208|3209440|self|-123|-123|-123|0.0|0.0|0.0|0.0|0.0|0.0|0.0" -H 'Content-Type: text/plain' 'http://localhost:9119/prediction'
It gives me this error
status":415,"error":"Unsupported Media Type","message":"Content type 'text/plain' not supported","path":"/prediction"
This is my controller class
import java.io.IOException; import java.util.HashMap; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.validation.annotation.Validated; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; @Validated @RestController public class MockController { @Autowired MockEndPoint mockendpoint; @Autowired MockConfig mockconfig; String w; String x; String y; String z; @RequestMapping(value = "/", method = RequestMethod.GET) public String index() { return "hello!"; } @RequestMapping(value = "/prediction", method = RequestMethod.POST, produces = {"application/json"},consumes= "text/html") public ResponseEntity<String> payloader1(@RequestBody HashMap<String,String> params ) throws IOException{ params = mockconfig.getHashmap(); if(params.containsKey(mockconfig.input1)) w = mockconfig.input1; String[] a = w.split("\\|"); if (a.length == 18) { return ResponseEntity.ok(params.get(mockconfig.input1)); } else { return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("Inccorect payload amount(18 parameters required"); } } }
This is my endpoint class
import java.io.File; import java.io.IOException; import java.nio.file.Files; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.util.ResourceUtils; @Configuration public class MockEndPoint { @Bean public String Payload1() throws IOException { File file = ResourceUtils.getFile("src/test/resources/Payload1.txt"); String content = new String(Files.readAllBytes(file.toPath())); return content; } @Bean public String Payload2() throws IOException { File file = ResourceUtils.getFile("src/test/resources/Payload2.txt"); String content = new String(Files.readAllBytes(file.toPath())); return content; } @Bean public String Payload3() throws IOException { File file = ResourceUtils.getFile("src/test/resources/Payload3.txt"); String content = new String(Files.readAllBytes(file.toPath())); return content; } @Bean public String Payload4() throws IOException { File file = ResourceUtils.getFile("src/test/resources/Payload4.txt"); String content = new String(Files.readAllBytes(file.toPath())); return content; } }
This is my config class
import java.io.IOException; import java.util.HashMap; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Configuration; @Configuration public class MockConfig { @Autowired MockEndPoint mockendpoint; String input1 = "ncs|56-2629193|1972-03-28|20190218|77067|6208|3209440|self|-123|-123|-123|0.0|0.0|0.0|0.0|0.0|0.0|0.0"; String input2 = "ncp|56-2629193|1955-11-28|20181213|73630|6404|182232|self|-123|-123|-123|0.0|0.0|0.0|0.0|0.0|0.0|33.35"; String input3 = "ncp|56-2629193|1955-11-28|20190103|73630|6404|182232|self|-123|-123|-123|0.0|0.0|0.0|0.0|0.0|0.0|33.35"; String input4 = "ncp|56-2629193|1955-11-28|20190213|73700|6404|182232|self|-123|-123|-123|0.0|20.0|325.0|0.0|0.0|269.28|269.28"; public HashMap<String,String> getHashmap() throws IOException { HashMap<String,String> hm = new HashMap<String,String>(); hm.put(input1,mockendpoint.Payload1()); hm.put(input2,mockendpoint.Payload2()); hm.put(input3,mockendpoint.Payload3()); hm.put(input4,mockendpoint.Payload4()); return hm; } }
-
Deadpool almost 5 years
@RequestBody
should be string type right? -
schooner_101 almost 5 yearsWould I have to create a hash map separately then from the requestBody?
-
Deadpool almost 5 yearsyes, create
HashMap
separately from that string -
fiveelements almost 5 yearsIn curl why don't you put -X POST? And the request payload does nor look like of type Map that you are expecting in controller method. What is the error you are getting after making it texr/ plain?
-
fiveelements almost 5 years@schooner_101 may I know why you changed the accepted answer? And why my answer does not solve your problem?
-
schooner_101 almost 5 years@fivelements I can't change the payload to JSON due to client standards. and ruhul s answer fixed it by changing it to plain in my code
-
fiveelements almost 5 years@schooner_101 did not I mention this in my answer:
The text/plain content won't be converted to Map by any converter. Otherwise, take request body as String and internally convert it to Map in your code.
-
schooner_101 almost 5 years@fiveelements you have a point. changed answer :)
-