How do I add or install the native x64 libraries for VLCJ?
Solution 1
you have to add "libvlc" and "libvlccore" dll path in your application. these will be present into your vlc installation folder. you can add following line of code to get it working.
NativeLibrary.addSearchPath("libvlc", "C:/VideoLAN/VLC");
here "C:/VideoLAN/VLC" is installation folder of vlc.
Solution 2
Make sure you are using either a straight x64 or x32 environment. This means:
- Windows x64 (obviously)
- Java JRE x64 (do not install a second x32 JRE)
- Java JDK x64
- VLC x64
Now you should be fine.
Solution 3
I read the vlcj instructions you posted.
It seems the vlcj library is using JNA and you can setup the library search path using the NativeLibrary class as is stated in the check program:
import uk.co.caprica.vlcj.binding.LibVlc;
import uk.co.caprica.vlcj.runtime.RuntimeUtil;
import com.sun.jna.Native;
import com.sun.jna.NativeLibrary;
public class Tutorial1A {
public static void main(String[] args) {
String vlcHome = "dir/with/dlls"; // Dir with vlc.dll and vlccore.dll
NativeLibrary.addSearchPath(
RuntimeUtil.getLibVlcLibraryName(), vlcHome
);
Native.loadLibrary(RuntimeUtil.getLibVlcLibraryName(), LibVlc.class);
}
}
Yo can try to run that code and check if it completes without exceptions.
vlcj instructions also points out that the architecture of the JRE is relevant; you should check your JRE architecture by typing:
java -version
The JRE architecture should match the VLC one (maybe you can check the VLC architecture in the About dialog). Both should be equal (32b or 64b).
However, it is strange that the error message refers to libvlc instead vlc or vlccore being executed in Windows.
Anyway, if adding the VLC path to the search path using NativeLibrary don't work and the JRE architecture matches the VLC one, you can add the code you are using to try to find out more.
Solution 4
This is how you load the vlc libraries using JNA:
NativeLibrary.addSearchPath(RuntimeUtil.getLibVlcLibraryName(), "Path to native library");
For my program I have the vlc "libvlc.dll" and "vlccore.dll" located in a sub-folder lib/VLC/
so I load the files relative to my "program.jar" using System.getProperty("user.dir")
and adding the path to the end:
NativeLibrary.addSearchPath(RuntimeUtil.getLibVlcLibraryName(), System.getProperty("user.dir") + "/lib/VLC");
If you want to load the library from the default VLC install path in windows 7 you can do it as follows:
NativeLibrary.addSearchPath(RuntimeUtil.getLibVlcLibraryName(), "C:/Program Files (x86)/VideoLAN/VLC");
Edit: If you run this code from within eclipse it will not work, unless you specify an absolute path to the VLC library files. If you want to test a relative path then first build the jar file and place it in the correct folder relative to the VLC library files.
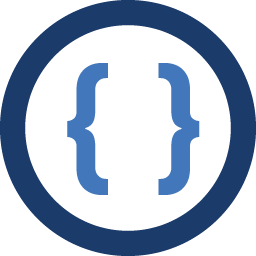
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
I am trying make a messenger program in Java that has a video player in it, so I'm using vlcj. But I receive this error:
Exception in thread "main" java.lang.UnsatisfiedLinkError:
Unable to load library 'libvlc':
The specified module could not be found.I have followed the tutorials from here, but I still get this error. This is the only error that I get.
I'm running on Windows 7 x64 Ultimate with a x64 VLC. I'm coding using the latest Eclipse version.
Can anyone guide me step-by-step on how to fix this?