How do I animate a scale css property using jquery?
Solution 1
You can perform the transition using CSS and then just use Javascript as the 'switch' which adds the class to start the animation. Try this:
$('button').click(function() {
$('.bubble').toggleClass('animate');
})
.bubble {
transform: scale(0);
width: 250px;
height: 250px;
border-radius: 50%;
background-color: #C00;
transition: all 5s;
}
.bubble.animate {
transform: scale(1);
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<button>Toggle</button>
<div class='col-lg-2 col-md-2 well bubble'></div>
Solution 2
Currently you cannot use animate
with the transform
property see here
However you can add a css transition
value and modify the css itself.
var scale = 1;
setInterval(function(){
scale = scale == 1 ? 2 : 1
$('.circle').css('transform', 'scale('+scale+')')
}, 1000)
.circle {
margin: 20px auto;
background-color: blue;
border-radius: 50%;
width: 20px;
height: 20px;
transform: scale(1);
transition: transform 250ms ease-in-out;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="circle"></div>
Solution 3
Better go with CSS3 Animations. For animation at a frequent interval you can use with browser supporting prefixes(-webkit,-moz,etc.)-
@keyframes fullScale{
from{
transform:scale(0);
}
to{
transform:scale(1);
}
}
.bubble:hover{
animation: fullScale 5s;
}
Refer this link- http://www.w3schools.com/css/css3_animations.asp
Or the above solution by @Rory is also a good way to addclass on an attached event.
Solution 4
Using the step callback and a counter. Here the counter is just the number 1.
'now' will be 0 to 1 over 5000ms.
$('.bubble').animate({transform: 1},
{duration:5000,easing:'linear',
step: function(now, fx) {
$(this).css('transform','scale('+now+')')}
})
Vanilla JS animate method (supported in modern browsers, no IE)
https://drafts.csswg.org/web-animations-1/#the-animation-interface
$('.bubble').get(0).animate([{"transform": "scale(1)"}],
{duration: 5000,easing:'linear'})
Solution 5
You can use Velocity.js. http://velocityjs.org/ For example:
$("div").velocity({
"opacity" : 0,
"scale" : 0.0001
},1800)
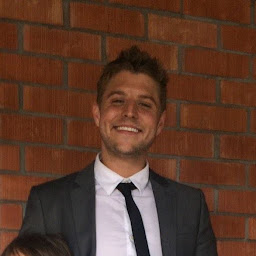
Weylin Wagnon
Updated on August 29, 2020Comments
-
Weylin Wagnon over 3 years
I am trying to have a circle div with the class of "bubble" to pop when a button is clicked using jQuery. I want to get it to appear from nothing and grow to its full size, but I am having trouble getting it to work. heres my code:
HTML Show bubble ... CSS
.bubble { transform: scale(0); }
Javascript
$('button').click(function(){ $('.bubble').animate({transform: "scale(1)"}, 5000, 'linear'); });