How do I concatenate 2 bytes?
37,578
Solution 1
It can be done using bitwise operators '<<' and '|'
public int Combine(byte b1, byte b2)
{
int combined = b1 << 8 | b2;
return combined;
}
Usage example:
[Test]
public void Test()
{
byte b1 = 0x5a;
byte b2 = 0x25;
var combine = Combine(b1, b2);
Assert.That(combine, Is.EqualTo(0x5a25));
}
Solution 2
Using bit operators:
(b1 << 8) | b2
or just as effective (b1 << 8) + b2
Solution 3
A more explicit solution (also one that might be easier to understand and extend to byte to int i.e.):
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Explicit)]
struct Byte2Short {
[FieldOffset(0)]
public byte lowerByte;
[FieldOffset(1)]
public byte higherByte;
[FieldOffset(0)]
public short Short;
}
Usage:
var result = (new Byte2Short(){lowerByte = b1, higherByte = b2}).Short;
This lets the compiler do all the bit-fiddling and since Byte2Short is a struct, not a class, the new does not even allocate a new heap object ;)
Solution 4
byte b1 = 0x5a;
byte b2 = 0x25;
Int16 x=0;
x= b1;
x= x << 8;
x +=b2;
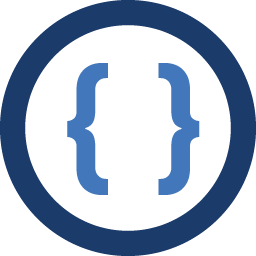
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have 2 bytes:
byte b1 = 0x5a; byte b2 = 0x25;
How do I get
0x5a25
? -
futureelite7 over 14 years(b1 << 8) + b2 will do the job faster.
-
paxdiablo over 14 years@futureelite7, that's unlikely to be the case with modern compilers - in any case, you should have used (b1<<8)|b2 :-)
-
paxdiablo over 14 yearsI'm pretty certain you didn't need to wrap that in a function :-) But you're right, so +1.
-
danatel over 14 yearsBeware of en.wikipedia.org/wiki/Endianness. It really matters what the bytes will be used for. Alhough most c# work is done on the Intel platform, the bytes may be send out as a part of network protocol where endiannes matters.
-
AK_ over 14 yearsoh the OR is nice :-) but it is still short... +1 for the OR :)
-
danielschemmel over 14 yearsmaybe - but if you want to express that something can be understood more than one way on a bit-wise level it is the tool of choice. Personally I prefer the shift+or method, but it becomes quite cumbersome when your target is i.e. a uint64, while the union still remains very readable in that case.
-
jason over 14 years@futureelite7: I note that
b1*256+b2
has one fewer character than(b1<<8)+b2
so actuallyb1*256+b2
is faster. :-) -
Jeppe Stig Nielsen over 11 yearsThere's also
BitConverter.ToUInt16(new[] { b2, b1, }, 0)
. -
scegg about 7 yearsFunc<byte, byte, int> combine; if (BitConverter.IsLittleEndian) { combine = new Func<byte, byte, int>((i, j) => j << 8 | i); } else { combine = new Func<byte, byte, int>((i, j) => i << 8 | j); } int result = combine(b1, b2);
-
siggi_pop over 5 yearsThis is ridiculous. Both are practically the same. running a trillion statments using: c = (a << 8) + b stopping timer @ 00:00:00.7638591 running a trillion statments using: c = a << 8 | b stopping timer @ 00:00:00.7634362 running a trillion statments using: c = a * 256 + b stopping timer @ 00:00:00.8592438 a: 1 00000000000000000000000000000001 b: 15 00000000000000000000000000001111 c: 271 00000000000000000000000100001111