How do I convert an integer value to decimal value in COBOL
Solution 1
COBOL provides several numeric representations. Some facilitate arithmetic operations, others facilitate presentation. It takes a while to sort them all out.
I think you may be looking for an implied decimal representation. The implied decimal is indicated by the letter 'V' in a PICture string for example:
WS-INT-NBR PIC 9(7).
WS-DEC-NBR PIC 9(5)V99.
Both of the above declarations contain 7 digits and occupy 7 bytes of storage. You can assign the value 1234567 to WS-INT-NBR as follows:
MOVE 1234567 TO WS-INT-NBR
but doing the same to WS-DEC-NBR would cause an overflow (truncation) because it can only hold 5 digits before an implied decimal point (the 'V'). However if you were to do the following:
MOVE 12345.67 TO WS-DEC-NBR
the actual contents of WS-DEC-NBR would be 1234567
(notice the decimal point
is gone and it contains a value equivalent to WS-INT-NBR). That is what is meant by implied
decimal point. COBOL 'knows' that WS-DEC-NBR has a decimal point between the
5th and 6th digits but does not actually store it. Any operations applied
to WS-DEC-NBR will take the implied decimal point into consideration.
Armed with this knowledge you can then make use of another COBOL feature, the REDEFINES declaration. REDEFINES tells the compiler to apply different data type processing rules to the same storage area. In one case you want to treat the memory area like an integer: PIC 9(7); and in other cases like an implied decimal value: PIC 9(5)V99). Do this as follows:
01.
02 WS-INT-NBR PIC 9(7).
02 WS-DEC-NBR REDEFINES WS-INT-NBR PIC 9(5)V99.
Now WS-INT-NBR and WS-DEC-NBR occupy the same memory area (the same 7 bytes). However when you reference WS-INT-NBR the integer representation is used. When you reference WS-DEC-NBR the decimal representation is used.
That gets us over the 'not dividing by 100' part of your problem. The next bit is displaying the decimal point when you need to. COBOL provides DISPLAY formats containing explicit 'punctuation' of which the decimal point is one. For example:
01 WS-DEC-DISPLAY PIC 9(5).99.
WS-DEC-DISPLAY contains an explicit decimal point within its PICture clause (do not confuse the period at the end of the line with the imbedded PICture clause decimal point). The content of WS-DEC-DISPLAY contains an explicit decimal point. So, to get the decimal point to display you need to MOVE something with an implied decimal point to it, as in:
MOVE WS-DEC-NBR TO WS-DEC-DISPLAY
if WS-DEC-NBR contained '1234567', WS-DEC-DISPLAY will contain '12345.67' after the MOVE.
The following program and displays put it all together for you:
IDENTIFICATION DIVISION.
PROGRAM-ID. EXAMPLE.
DATA DIVISION.
WORKING-STORAGE SECTION.
01.
02 WS-INT-NBR PIC 9(7).
02 WS-DEC-NBR REDEFINES WS-INT-NBR PIC 9(5)V99.
01 WS-DEC-DISPLAY PIC 9(5).99.
PROCEDURE DIVISION.
MOVE 1234567 TO WS-INT-NBR
MOVE WS-DEC-NBR TO WS-DEC-DISPLAY
DISPLAY 'WS-INT-NBR : ' WS-INT-NBR
DISPLAY 'WS-DEC-NBR : ' WS-DEC-NBR
DISPLAY 'WS-DEC-DISPLAY: ' WS-DEC-DISPLAY
ADD +1 TO WS-INT-NBR
MOVE WS-DEC-NBR TO WS-DEC-DISPLAY
DISPLAY 'INT-NBR PLUS 1: ' WS-DEC-DISPLAY
ADD +1 TO WS-DEC-NBR
MOVE WS-DEC-NBR TO WS-DEC-DISPLAY
DISPLAY 'DEC-NBR PLUS 1: ' WS-DEC-DISPLAY
GOBACK
.
Output:
WS-INT-NBR : 1234567
WS-DEC-NBR : 1234567
WS-DEC-DISPLAY: 12345.67
INT-NBR PLUS 1: 12345.68 <= notice which digit incremented
DEC-NBR PLUS 1: 12346.68 <= notice which digit incremented
Solution 2
Redefine your value using an implied decimal. E.G.
10 ws-integer pic 9(7) value 1234567.
10 ws-decimal redefines ws-integer pic 9(5)v99.
When you refer to this as ws-decimal, you will get 12345.67.
So if you move something to ws-integer, you can access it with the decimals in the correct position using the other field.
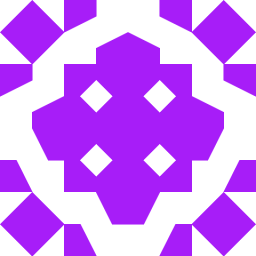
user1053713
Updated on July 13, 2022Comments
-
user1053713 11 months
How do I convert an integer value to decimal value in COBOL?
I need to display 1234567 as 12345.67
I cannot divide the variable by 100.
-
user1053713 over 11 yearsi tried that, however after doing this i m getting vale as 34567.00
-
Joe Zitzelberger over 11 yearsYou must be doing a "Move ws-integer to ws-decimal" you need to redefine the data area.
-
Raja Reddy over 11 yearsThats a worthy explanation NealB.
Hates off to your patience