How do I get the user name of the current user?
Solution 1
#include <stdio.h>
int main(void)
{
printf("%s\n", getenv("USERPROFILE")); // Print user's home directory.
return 0;
}
To get the user name instead of the home path replace USERPROFILE
with USERNAME
.
Solution 2
What you are looking for, here, is probably more SHGetKnownFolderPath
. The function lets you find per-user special folders. This is preferred to querying usernames because the home folder may not have the same name as the user.
WSTR* location;
HRESULT hr = SHGetKnownFolderPath(FOLDERID_RoamingAppData, 0, NULL, &location);
if (SUCCEEDED(hr))
{
// location contains the folder path
// call CoTaskMemFree to free up the memory once you're done with it
CoTaskMemFree(location);
}
The list of so-called known folders is available here.
Solution 3
The function to get user name on windows is GetUserName
This answer, probably, will help you too.
Solution 4
you could use the following code to get the Username.
#include <stdlib.h>
void main(void)
{
//following gets the appdata folder
char szAppData[1024];
char * szBufer = 0;
szBufer = getenv ("APPDATA");
if (szBufer != NULL)
{
strcpy(szBufer , szAppData);
}
//following code gets the user name
char szOSUserName[1024];
szBufer = getenv ("USERNAME");
if (szBufer != NULL)
{
strcpy(szBufer , szOSUserName);
}
}
Solution 5
You can get the name of the current user with GetUserName
:
#include <Windows.h>
#include <Lmcons.h>
#include <stdio.h>
int main()
{
char name[UNLEN + 1];
DWORD cch = UNLEN + 1;
if (GetUserName(name, &cch))
{
char cmd[100 + UNLEN + 1];
sprintf(cmd, "echo The username is \"%s\"", name); // Silly demo command
system(cmd);
}
return 0;
}
Use GetUserNameEx
if you want the name in a specific format.
If you need to get the path to a special folder like "My Documents" or "Desktop" you should use the special folder functions like SHGetFolderPath
or SHGetKnownFolderPath
.
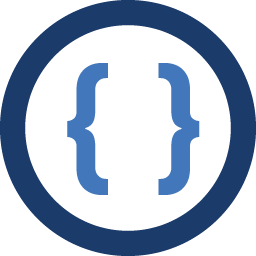
Admin
Updated on July 11, 2022Comments
-
Admin almost 2 years
I want to access the user name in the Windows using C programming and use that name to create the path to the particular file like "c:\users\john\Roaming.....and so on". So for every system user name e.g "john" is different. Help me to find the user name at run time.