How long does a UDP packet stay at a socket?
Solution 1
Normally, the data will be buffered until it's read. I suppose if you wait long enough that the driver completely runs out of space, it'll have to do something, but assuming your code works halfway reasonably, that shouldn't be a problem.
A typical network driver will be able to buffer a number of packets without losing any.
Solution 2
If data is sent to the client but the client is busy executing something else, how long will the data be available to read using
recvfrom()
?
Forever, or not at all, or until you close the socket or read as much as a single byte.
The reason for that is:
UDP delivers datagrams, or it doesn't. This sounds like nonsense, but it is exactly what it is.
A single UDP datagram relates to either exactly one or several "fragments", which are IP packets (further encapsulated in some "on the wire" protocol, but that doesn't matter). The network stack collects all fragments for a datagram. If the checksum on any of the fragments is not good, or any other thing that makes the network stack unhappy, the complete datagram is discarded, and you get nothing, not even an error. You simply don't know anything happened.
If all goes well, a complete datagram is placed into the receive buffer. Never anything less, and never anything more. If you try to recvfrom
later, that is what you'll get.
The receive buffer is obviously necessarily large enough to hold at least one max-size datagram (65535 bytes), but since usually datagrams will not be maximum size, but rather something below 1280 bytes (or 1500 if you will), it can usually hold quite a few of them (on most platforms, the buffer defaults to something around 128-256k, and is configurable).
If there is not enough room left in the buffer, the datagram is discarded, and you get nothing (well, you do still get the ones that are already in the buffer). Again, you don't even know something happened.
Each time you call recvfrom
, a complete datagram is removed from the buffer (important detail!), and you get up to the number of bytes that you requested. Which means if you naively try read a few bytes and then a few bytes again, it just won't work. The first read will discard the rest of the datagram, and the subsequent ones read the first bytes of some future datagrams (and possibly block)!
This is very different from how TCP works. Here you can actually read a few bytes and a few bytes again, and it will just work, because the network layer simulates a data stream. You give a crap how it works, because the network stack makes sure it works.
Also, what happens if a second packet is sent before the first one is read, is the first one lost and the next one sitting there waiting to be read?
You probably meant to say "received" rather than "sent". Send and receive have different buffers, so that would not matter at all. About receiving another packet while one is still in the buffer, see the above explanation. If the buffer can hold the second datagram, it will store it, otherwise it silently goes * poof *.
This does not affect any datagrams already in the buffer.
Solution 3
If data is sent to the client but the client is busy executing something else, how long will the data be available to read using recvfrom()?
This depends on the OS, in windows, I believe the default for each UDP socket is 8012, this can be raised with setsockopt() Winsock Documentation So, as long as the buffer isn't full, the data will stay there until the socket is closed or it is read.
Also, what happens if a second packet is sent before the first one is read, is the first one lost and the next one sitting there wating to be read?
If the buffer has room, they are both stored, if not, one of them gets discarded. I believe its the newest one but I'm not 100% Sure.
Related videos on Youtube
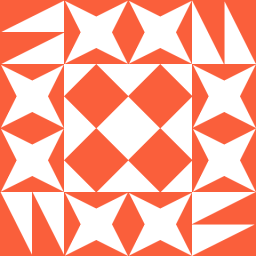
T.T.T.
Updated on June 04, 2022Comments
-
T.T.T. 5 months
If data is sent to the client but the client is busy executing something else, how long will the data be available to read using recvfrom()?
Also, what happens if a second packet is sent before the first one is read, is the first one lost and the next one sitting there wating to be read?
(windows - udp)
-
millimoose about 11 yearsOnce the data actually reaches the target machine, I'd assume it's probably treated similarly to TCP traffic – the datagram will sit in a buffer somewhere, in the order in which the datagrams arrived, until it's read. (A full buffer might cause the OS to drop the packet altogether though.)
-
Remus Rusanu about 11 yearsIf you're worried about packet loss and order, why do you use UDP? If you find yourself asking such questions is a very strong indication you should be doing TCP
-
-
nos about 11 yearsIt'll fill up the socket buffer mainly, not (just) the driver. It'll stay in the socket buffer until you read it or the process exits. Once the socket buffer is full, new packets arriving is dropped.
-
Ben Voigt over 6 years"You simply don't know anything happened" is not precisely true, on some stacks there are statistics concerning packets discarded due to missing fragments, invalid checksums, insufficient buffer space, etc. But it is true that you never get partial packets delivered using the usual mechanism.
-
Darkgaze over 1 yearBut this is not true. I sent packages to a socket, then read the socket after a second, and there was nothing there. If I read it actively (rcvfrom) in a loop with non-blocking calls, I get packages. How come this happens?