Jest has detected the following 1 open handle potentially keeping Jest from exiting
11,780
Solution 1
you need to call done()
in the end()
method
const request = require("supertest");
const app = require("../app");
let server = request(app);
it("should return 404", done =>
server
.get("/")
.expect(404)
.end(done);
});
Solution 2
This trick worked;
afterAll(async () => {
await new Promise(resolve => setTimeout(() => resolve(), 500)); // avoid jest open handle error
});
As described in this github issue.
Related videos on Youtube
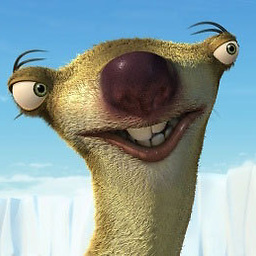
Comments
-
shellakkshellu about 4 hours
Here are my HTTP routes
app.get('/', (req, res) => { res.status(200).send('Hello World!') }) app.post('/sample', (req, res) => { res.status(200).json({ x:1,y:2 }); })
I would like to test for the following
1)
GET
request working fine.2)the
/sample
response contains the properties andx
andy
const request = require('supertest'); const app = require('../app'); describe('Test the root path', () => { test('It should response the GET method', () => { return request(app).get('/').expect(200); }); }) describe('Test the post path', () => { test('It should response the POST method', (done) => { return request(app).post('/sample').expect(200).end(err,data=>{ expect(data.body.x).toEqual('1'); }); }); })
But I got the following error on running the test
Jest has detected the following 1 open handle potentially keeping Jest from exiting:
return request(app).get('/').expect(200);
-
shellakkshellu about 4 yearsTried your answer but i got this error
Jest did not exit one second after the test run has completed
-
Shraddha Goel about 4 yearsYou can go throw the docs there are several methods. Actually what is needed at your point is the way of calling. I have never used return request(app) in Jest Testing. I mainly used expect(To make call function).toEqual(To test its value) and it works perfectly fine for me
-
shellakkshellu about 4 yearsI am asking about the HTTP response from the http post call